Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial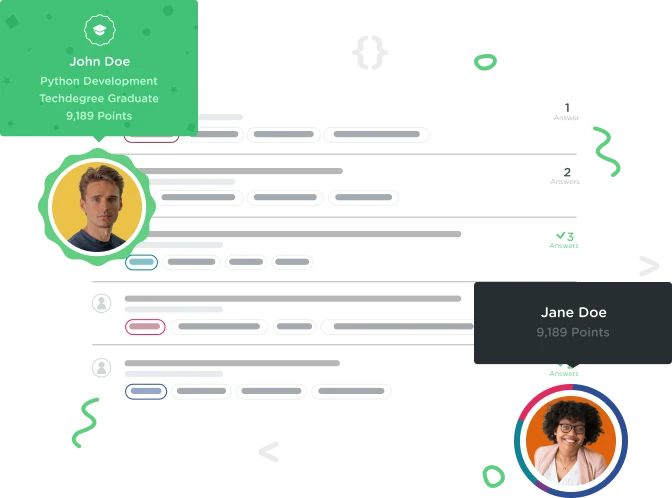

Gokul Nishanth
Courses Plus Student 581 PointsHow to finish this challenge?
And, finally, if I have doubles, I want to reroll the hand. Add a classmethod to CapitalismHand named reroll that returns a new instance of the class, effectively rerolling the hand.
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self):
super().__init__(size=2, die_class=D6)
@property
def doubles(self):
if len(self._by_value(1)) == 2:
reroll()
if len(self._by_value(2)) == 2:
reroll()
if len(self._by_value(3)) == 2:
reroll()
if len(self._by_value(4)) == 2:
reroll()
if len(self._by_value(5)) == 2:
reroll()
if len(self._by_value(6)) == 2:
reroll()
else:
return False
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
def reroll(self):
ch = CapitalismHand()
return ch
6 Answers
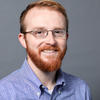
Kurt Maurer
16,725 PointsHey Gokul,
I was having trouble with this one as well. Your reroll()
method works perfectly, but you need to add the @classmethod
above it. Additionally, I would consider refactoring your doubles()
method, as the challenge never asked us to call this method in the event that we get doubles, and I wonder if it may be affecting your results. Since a Hand
is a list of two objects, all you have to do is compare them to each other to get a True
or False
.

Erika Suzuki
20,299 PointsComing from Java. This is by far the hardest OOP lesson I ever studied.

Benyam Ephrem
16,505 PointsHahahaahahaha, same here. Went from Java to JS and it was a breeze but this is certainly challenging. But I guess it is the same as with anything new you learn. Over time it becomes natural
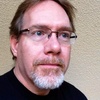
Chris Freeman
Treehouse Moderator 68,441 PointsBenyam, I hope you mean "over time" vs "overtime". Though "Overtime: it becomes natural" is not an inaccurate statement! ?

Benyam Ephrem
16,505 PointsI apologize...I've been coding overtime over time so my fingers are typing faster than my brain can think. Corrected it :)

Paul Bentham
24,090 PointsCheckout this Stack Overflow answer: http://stackoverflow.com/questions/13260557/create-new-class-instance-from-class-method

Ankoor Bhagat
1,097 Points[Solution redacted]

Khaleel Yusuf
15,208 PointsI copied and pasted Murat Mermerkaya and fixed the mistake but I said: "Try again"

Khaleel Yusuf
15,208 PointsI meant Murat Mermerkaya's code
Murat Mermerkaya
2,346 PointsMurat Mermerkaya
2,346 PointsI did like your comment but still not working:
[MOD: added ```python formatting -cf]
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsSpecifically, by using
reroll()
within thedoubles()
method it will raise an error since "reroll()" is not defined. Properly, it would beself.reroll()
. But refactoring is the better approach. You can directly compare the values of the two die using :self[0].value == self[1].value
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsMurat Mermerkaya, your
doubles
method is missing areturn
statement.Radovan Valachovic
Python Web Development Techdegree Graduate 20,368 PointsRadovan Valachovic
Python Web Development Techdegree Graduate 20,368 PointsHello Kurt, why you saying "as the challenge never asked us to call this method in the event that we get doubles" - the challenge is specifically asking - "And, finally, IF I HAVE DOUBLES, I want to reroll the hand.".... it asks in the case of double only then reroll the hand...