Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial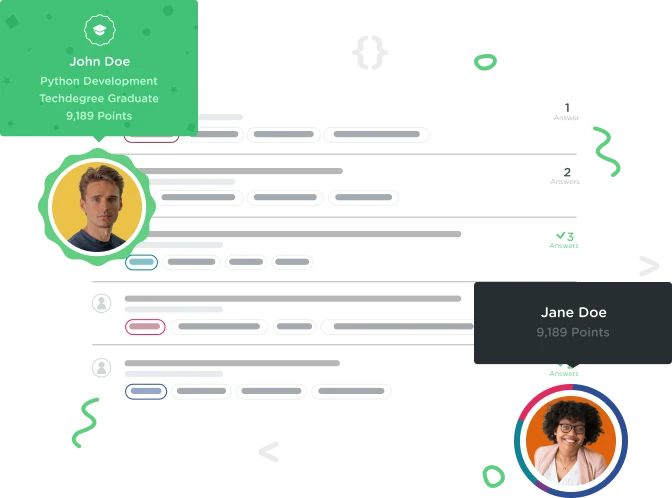

Kenneth Phillips
Courses Plus Student 10,188 PointsHow to get an int value from a void method?
I have an app where if the user gets a question right in the box then it will add a point. After the actual game activity finishes I launch a new activity where they can submit their score. I need to get the point value from the previous activity but I can't because the whole checkAnswer() returns void. How would I get the point value over to my other activity?
public class GameActivity extends AppCompatActivity {
private Game game;
private ImageView gameImageView;
private TextView gameTextView;
private Button submitButton;
private TextView pointsTextView;
private int points;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_game);
gameImageView = (ImageView) findViewById(R.id.gameImageView);
gameTextView = (TextView) findViewById(R.id.gameTextView);
pointsTextView = (TextView) findViewById(R.id.pointsTextView);
submitButton = (Button) findViewById(R.id.submitButton);
game = new Game();
loadPage(0);
checkAnswer(0);
}
private void loadPage(final int pageNumber) {
final Page page = game.getPage(pageNumber);
if (page.isFinalPage()) {
startSubmit();
}
Drawable image = ContextCompat.getDrawable(this, page.getImageId());
gameImageView.setImageDrawable(image);
String pageText = getString(page.getTextId());
gameTextView.setText(pageText);
final int nextPage = page.getNextPage();
submitButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
loadPage(nextPage);
checkAnswer(nextPage);
}
});
}
private void checkAnswer(int pageNumber) {
final Page page = game.getPage(pageNumber);
String gameAnswer = page.getAnswer();
String gameEditText = ((EditText) findViewById(R.id.gameSquare)).getText().toString();
if (gameEditText.equals(gameAnswer))
points++;
String pointsText = String.format("%1$s", points);
pointsTextView.setText(pointsText);
}
private void startSubmit() {
Intent submitIntent = new Intent(this, SubmitActivity.class);
startActivity(submitIntent);
}
public int getPoints() {
return points;
}
}
4 Answers

Kenneth Phillips
Courses Plus Student 10,188 PointsIn my other activity I'm trying to retrieve this value but the app crashes.
public TextView finalScoreTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_submit);
String points = getIntent().getStringExtra("POINTS");
finalScoreTextView = (TextView) findViewById(R.id.scoreTextView);
String text = String.format("Score: %1$s", points);
finalScoreTextView.setText(text);
}
Do you see a problem?

Ben Deitch
Treehouse TeacherHey Kenneth! You can pass the 'points' field to the other Activity inside the Intent:
private void startSubmit() {
Intent submitIntent = new Intent(this, SubmitActivity.class);
submitIntent.putExtra("POINTS", points);
startActivity(submitIntent);
}

Kenneth Phillips
Courses Plus Student 10,188 PointsIt throws a FileNotFound error almost 20 times. Other than that it won't tell me the issue. It did this before and a moderator told me not to worry about it.

Ben Deitch
Treehouse TeacherGotcha. If it's something you can move past, 'don't worry about it' is usually good advice :)

Kenneth Phillips
Courses Plus Student 10,188 PointsAlright well it appears maybe this a problem with my IDE or something got corrupted. Thanks for the help anyway.
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherWhat error does it show?