Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial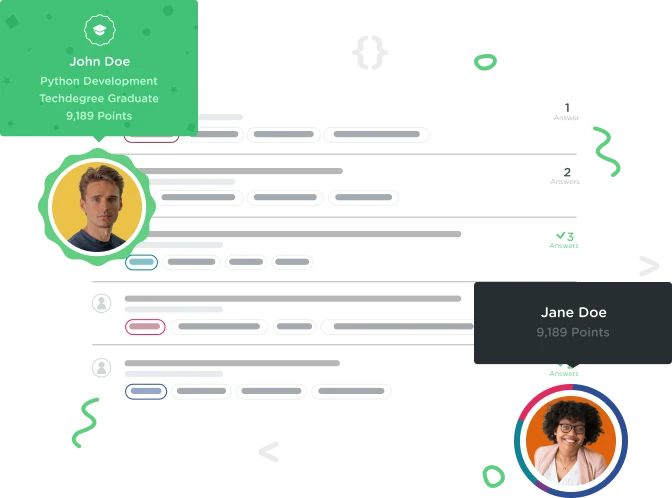

Tom Finet
7,027 PointsHow to get firebase user token from anywhere in an app?
When a user signs up with firebase in my android app, a FirebaseUser object is generated and from this I retrieve the user token to save as a unique identifier for each user in my google cloud endpoints backend.
I use an auth state listener which is called when the user signs up:
mAuthListener = new FirebaseAuth.AuthStateListener() {
@Override
public void onAuthStateChanged(@NonNull FirebaseAuth firebaseAuth) {
FirebaseUser user = firebaseAuth.getCurrentUser();
if (user != null) {
mUserId = user.getToken(true).toString();
SignUpAboutFragment signUpAboutFragment = new SignUpAboutFragment();
FragmentTransaction ft = getSupportFragmentManager().beginTransaction();
ft.replace(R.id.sign_up_fragment_container, signUpAboutFragment, SIGN_UP_ABOUT_FRAGMENT_TAG);
ft.addToBackStack(null);
ft.commit();
}
}
};
The field mUserId is then used to save the profile into the google datastore:
mPresenter.saveUserData(firstName, lastName, birthday, mUserId, this, MainActivity.class); In a different activity I also wish to use the current users token to make calls to my endpoint api:
//Different activity to the above.
FirebaseUser user = FirebaseAuth.getInstance().getCurrentUser();
if (user != null) {
// Using getToken as recommended in the firebase docs.
mUserId = user.getToken(true).toString();
}
When I log both mUserId fields in the 2 activities I find that they are different.
Why is mUserId changing and how do I retrieve the same token of the current user from anywhere in my app?