Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial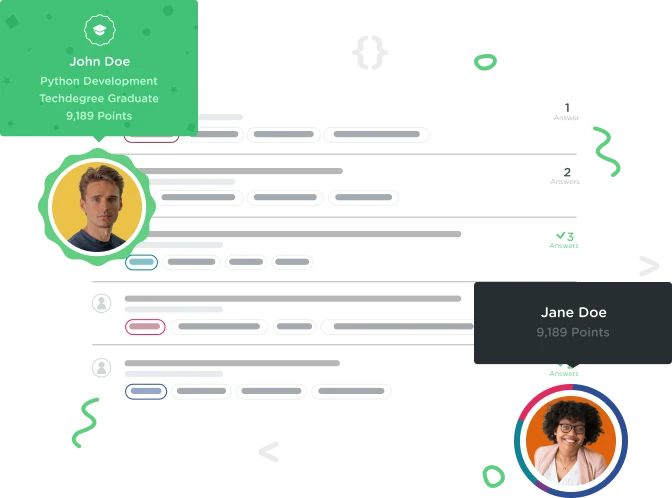
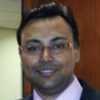
Abhijit Das
5,022 PointsHow to get previous object of the last item from the array list?
Hello friends, i want to retrieve the previous item from the last item of a arraylist. I tried the pop method but i am getting the last one, not the previous item of the last item. As an example if I add into my arraylist by input red,green,blue etc. After clicking the previous color btn I want green and after another click Red not blue first. How could i achive this. Pls help me.Thank you. https://codepen.io/pen/?editors=1010
3 Answers
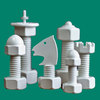
Steven Parker
229,732 PointsYou can do that with the splice method:
In this case: "var secondlast = colors.splice(colors.length - 2, 1)[0];
"
You could also create your own "pop2" method to work like "pop" but take the second-last item:
Array.prototype.pop2 = function () {
if (this.length < 2) return;
return this.splice(this.length - 2, 1)[0];
}
FYI: your link just goes to an empty codepen.
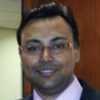
Abhijit Das
5,022 PointsThank you Steven Parker, you always help us whenever we stuck or lost in JavaScript Void..:) Thank you. "FYI: your link just goes to an empty codepen." - that's really a silly mistake from me. Sorry. Now i need another help. I want to get the next element. Suppose i add red,green,blue etc. color in my array list by using input.value. Once i click the previous color btn, getting now right element respectively such as green,red. Thanks for showing me the splice method(). Now once i click next color btn i want to get next color like respectively red,green etc. can you show me how can i get that result. I tried push method but the next btn doesn't showing any result. Thanks in advance. https://codepen.io/anon/pen/NgMOMX?editors=1010
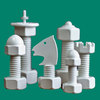
Steven Parker
229,732 PointsIt's not clear to me what you want the "next color" button to do. But here's some observations:
- "objCol" doesn't exist in the scope of the "next button" handler — did you mean to make it global?
- you could use a plain "pop" instead of using slice with those arguments.
- instead of doing "push" followed by "pop" you could skip both and use the variable directly
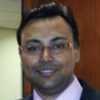
Abhijit Das
5,022 PointsHi Steven,
I just want to create a color changing h1 element. My goal is simple, user can change header element color by input different color values and also can get previous or next color by clicking on previous and next color buttons. It's only for learning.
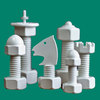
Steven Parker
229,732 PointsWhat I mean is I'm not sure what the word "next" refers to. I thought the "next" one would be the one you enter and then hit "add".
Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsAakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsHey Steven , I didn't understand why you have put
[0]
at the end just before semicolon in your example. I have always used[0]
only with "Array" name. But here how it is used and what it is doing?"var secondlast = colors.splice(colors.length - 2, 1)[0];"
And my second question is -
"Is it necessary to write within
this.splice()
function?return this.splice(this.length - 2)[0];
Is it incorrect?Is this only return the second element and will not delete it?
Steven Parker
229,732 PointsSteven Parker
229,732 PointsThe "splice" method returns an array, and then the "[0]" gets the first element from it.
And "splice" does delete the selected element(s) from the array you use it on.
Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsAakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsCan we write
pop
function as-Is it correct?
Steven Parker
229,732 PointsSteven Parker
229,732 PointsIf you want to create a function instead of an Array method, you'll need to replace "this" with your parameter name ("accArray"). Also, it looks like your function is missing the final closing brace (}). And you might want to allow it to work on arrays of exactly 2 items in addition to the longer ones.
And the name "pop" might be confusing — I called the Array method "pop2" to imply that it was removing the 2nd last item instead of the last.