Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial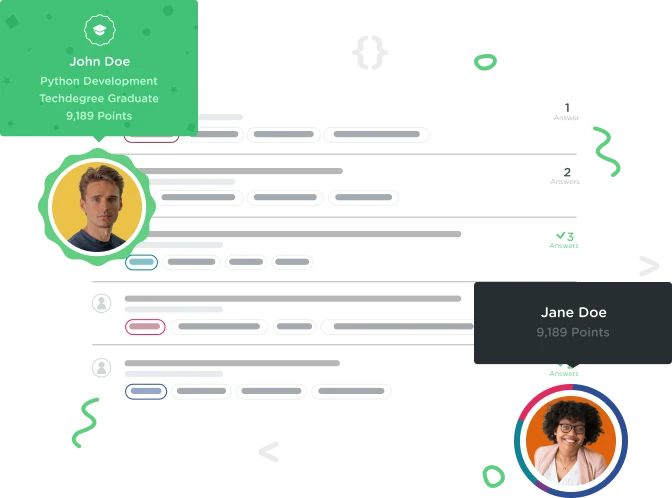

rl8
1,021 PointsHow to get selected option from three different dropdown option elements?
I'm trying to make a generator and if the user selects certain options on the three different dropdown menus it will show a hidden div. I was testing it with an alert first and no matter what options are picked it still throws up the alert. Below is my code (apologies if it is not great i'm still very new to coding)
window.onload = function(){
let generate = document.getElementById("mybutton").onclick = function(){
var select = document.getElementById('opt1');
var select2 = document.getElementById('opt2');
var select3 = document.getElementById('opt3');
var selectTest = select.selected;
var selectTest2 = select2.selected;
var selectTest3 = select3.selected;
var show = document.getElementById('hidden');
if (selectTest === "Grass1"); {
if (selectTest2 === "Grass2"); {
if (selectTest3 === "Empty3"); {
}
}
}
alert("fire");
}
};
2 Answers
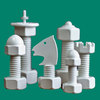
Steven Parker
230,274 PointsThese conditionals are all nested instead of being peers. Use "else" to make sure only one conditional (or the final alert) gets used. Also note that the variables are all assigned to Boolean element attributes, so they should be tested directly instead of comparing them to strings:
if (selectTest) {
// handle first choice
}
else if (selectTest2) {
// handle second choice
}
else if (selectTest3) {
// handle third choice
}
else {
alert("fire");
}
And there should not be a semicolon between an "if" conditional and the brace beginning the code block.

rl8
1,021 PointsHi, here is my whole project, I did some googling to try and get answers but I'm still no close to figuring out what is wrong. when certain options are selected I want it to show a hidden div, for example, if Grass, Grass and N/A are selected from all three different dropdown menus I want it to show the div. I have been testing it with an alert to see if it works hence why there is an alert.
any help is much appreciated, thanks
<body>
<div class="main-wrapper">
<header>
<nav class="nav-bar">
<ul>
<li class="current"><a href="index.html">Home</a></li>
<li><a href="about.html">About</a></li>
<li><a href="links.html">Links</a></li>
</ul>
</nav>
</header>
<section>
<div class="inner-wrapper">
<h1 class="title">Type Matchup</h1>
<div class="types">
<div class="type-1">
<h3>Primary Type</h3>
<select id="opt1" name="primarytype" id="primary-type">
<option id="Grass1" value="1">Grass</option>
<option class="test" value="2" >Fire</option>
<option class="test" value="3">Water</option>
<option class="test" value="4">Electric</option>
<option class="test" value="5">Psychic</option>
<option class="test" value="6">Fighting</option>
<option class="test" value="7">Dark</option>
<option class="test" value="8">Steel</option>
<option class="test" value="9">Fairy</option>
<option class="test" value="10">Dragon</option>
</select>
</div>
<div class="type-2">
<h3>Secondary Type</h3>
<select id="opt2" name="Primary-Type" id="secondary-type">
<option id="Grass2" value="11">Grass</option>
<option class="test" value="12">Fire</option>
<option class="test" value="13">Water</option>
<option class="test" value="14">Electric</option>
<option class="test" value="15">Psychic</option>
<option class="test" value="16">Fighting</option>
<option class="test" value="17">Dark</option>
<option class="test" value="18">Steel</option>
<option class="test" value="19">Fairy</option>
<option class="test" value="20">Dragon</option>
<option class="test" value="21">N/A</option>
</select>
</div>
<div class="type-3">
<h3>Tera Type</h3>
<select id="opt3" name="Primary Type" id="tera-type">
<option class="test" value="22">Grass</option>
<option class="test" value="23">Fire</option>
<option class="test" value="24">Water</option>
<option class="test" value="25">Electric</option>
<option class="test" value="26">Psychic</option>
<option class="test" value="27">Fighting</option>
<option class="test" value="28">Dark</option>
<option class="test" value="29">Steel</option>
<option class="test" value="30">Fairy</option>
<option class="test" value="31">Dragon</option>
<option class="test" value="32">N/A</option>
</select>
</div>
<div>
</div>
</section>
<button id="mybutton" onclick="validate()">Gen</button>
<div id="hidden" style="display: none;">Water</div>
</div>
</div>
</body>
function validate(){
var primeType = document.getElementById('opt1');
var primeTypeValue = primeType.options[primeType.selectedIndex].value;
var subType = document.getElementById('opt2');
var subTypeValue = subType.options[subType.selectedIndex].value;
var teraType = document.getElementById('opt3');
var teraTypeValue = teraType.options[teraType.selectedIndex].value;
if (primeTypeValue === '1') {
}
else if (subTypeValue === '11') {
}
else if (teraTypeValue === '32') {
}
else{
alert("fire");
}
}
rl8
1,021 Pointsrl8
1,021 PointsI've changed it and it's still showing the alert even though i only want it to show the alert when certain options on the dropdown lists have been selected
Steven Parker
230,274 PointsSteven Parker
230,274 PointsCan you be more explicit about the intended function? I thought you wanted the alert to show only when none of the options had been selected.
Also, if you program this function to run "onload", then it will run before the user has a chance to make any selection. You will probably want to change the event so it only runs when a selection has been made.
rl8
1,021 Pointsrl8
1,021 PointsI have three dropdown menus that have ten options each, I want to output a hidden div if certain options have been picked by the user. I was testing it with an alert first.
Thanks for your replies, much appreciated.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsAs I said, I misunderstood the objective and the first suggestion would show the alert only if none of the selections were made. If you want to act when all the choices are made instead, and reveal the hidden div instead of an alert, you might do this:
Your original idea of nesting the tests would also have worked if you had placed the alert inside the deepest level. Combining the tests with the logic operators is a bit more efficient and possibly more clear on reading.