Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial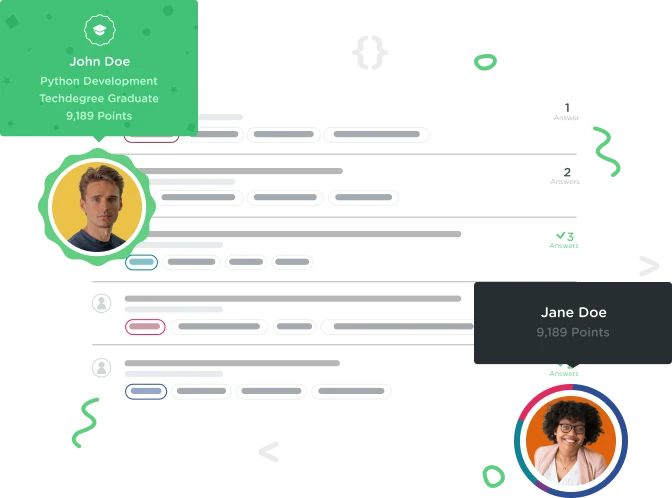
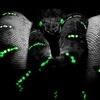
Robert Peters
3,728 PointsHow to get user input, then use that string as the name of a new variable? globals(), setattr() or other?
I've got a function that creates a list that is named from user input:
all_lists = ['games', 'shows', 'cars']
def add_list():
new_list_name = input("Name of list:\n>>>")
if new_list_name:
all_lists.append(new_list_name)
globals()[new_list_name] = []
print("SUCCESS! List named '{}' has been created.".format(new_list_name))
else:
print("FAILED! Nothing entered for name of list!")
And I've got a function that shows a list based on user input:
def show_list():
list_to_show = input("Name of list: ")
# check if list in in all_lists
if list_to_show in all_lists:
# check if the list called is empty
if globals()[list_to_show]: # this works, but if I use 'if globals()[list_to_show] is True:' instead it always returns False instead of True. Why is this? Am I using 'is True' incorrectly?
# print out each item in the list
for item in globals()[list_to_show]:
print(item)
Also, am I using globals() correctly, or is there a better way? I was looking for a way to create a list that's named from the string returned from user input, and globals()[variable_from_input] = [] was all I could find suggested online other than using a 'dictionary', and I've no idea how to use either correctly. I think I'm doing a few things wrong but I can't think what. Total noob sorry, Just started the python track!
1 Answer

Kamil Borowski
8,400 PointsThe 'is' operator compares the identity of two objects while the '==' operator compares the values of two objects. Instead of "if globals()[list_to_show] is True:" use "if globals()[list_to_show] == True:" but this is the same as "if globals()[list_to_show]:"