Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial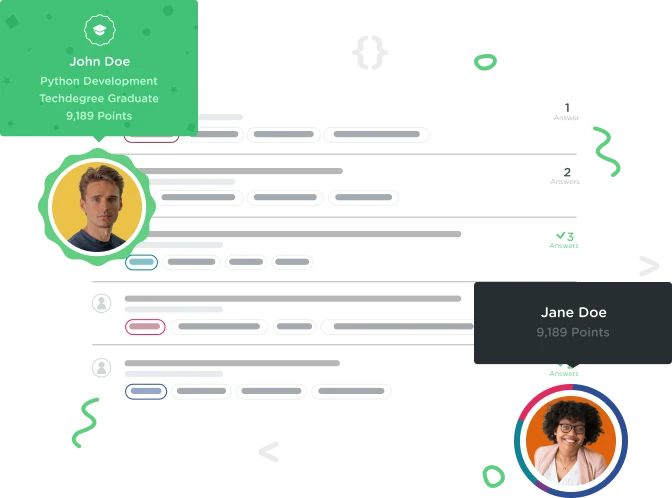
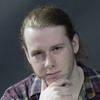
Marco Koopman
5,290 PointsHow to global scope a var in a class
Hey!
I'm wondering how you can make a variable global ( so that I don't have to use 'self').
Normally it works to define it right under the class and above the constructor but in Python this gives me a scoping error.
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(length):
laps += 1
self.fuel_remaining = self.length * 0.125
2 Answers
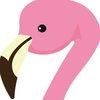
Dave StSomeWhere
19,870 PointsI think your issue is that you are missing self in your run_lap() method def - not any issue with scoping.
The scoping seems fine (as you described) if the following code is what you meant:
class RaceCar:
test_var = "hello Race Car"
test2 = "Gonna assign to instance"
def __init__(self, color, fuel_remaining, **kwargs):
self.laps = 0
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining -= length * 0.125
self.laps += 1
test_var = "changed in runlap"
self.test2 = "Assigned to Instance***"
print("test_var in runlap ==> " + test_var)
print("self.test2 in runlap ==> " + self.test2)
a = RaceCar("Blue", 100)
a.run_lap(25)
print("fuel remaining ==> " + str(a.fuel_remaining))
print("test var ===> " + a.test_var)
print("test2 ===> " + a.test2)
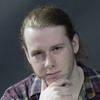
Marco Koopman
5,290 PointsHey!
I've ajusted the code and it doesn't give any errors anymore. However the fuel_remaining variable isn't changing. I really don't understand how this scoping works.
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
RaceCar.laps += 1
self.fuel_remaining = length * 0.125
print(self.fuel_remaining)
Marco Koopman
5,290 PointsMarco Koopman
5,290 PointsHey! Thanks for the reply. I already fixed the missing self parameter but I still get an error saying the variable 'laps' is referenced before assignment
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsYes, I see what you are talking about - laps doesn't exist without any reference. It need to be either
self.laps
for the instance orRaceCar.laps
for the class. Justlaps
doesn't exist and attemptinglaps += 1
results in an error within the method.