Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial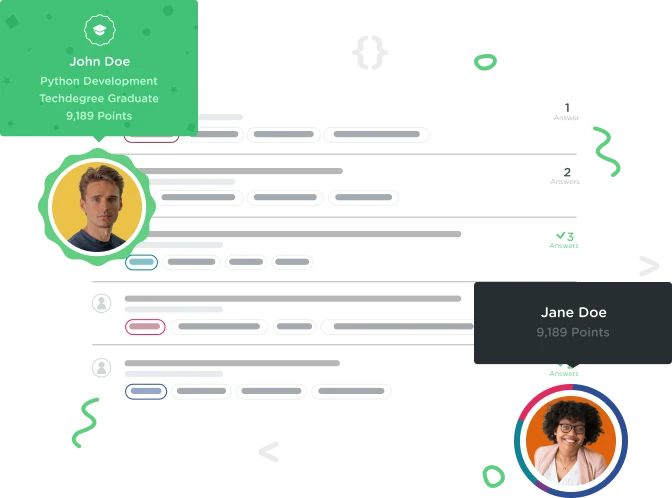

Marc Norris
625 PointsHow to handle 2 exceptions in one Try block
Hi there, I am trying to have the Try/Except block that we created handle 2 conditions and return 2 possible inputs for the user to correct their mistakes
As it stands it seems to only return the "Please enter a numerical value error", ignoring the tickets_remaining exception and also restarts the code rather than continuing.
Can anyone help me understand what I need to change?
TICKET_PRICE = 10
SERVICE_CHARGE = 2
tickets_remaining = 100
# Create the calculate_price function. It takes number of tickets and returns
# int(quantity) * TICKET_PRICE
def calculate_price(quantity):
# Create a new consant for the $2 service charge
# Add the service charge to calculate_price
return (int(quantity) * TICKET_PRICE) + SERVICE_CHARGE
while tickets_remaining >= 1:
user_name = input("What is your name? ")
print("Hi {}! There are currently {} tickets remaining.".format(user_name, tickets_remaining))
desire_to_buy = input("Would you like to buy any? ")
if desire_to_buy.lower() == "yes":
quantity = (input("Great! How many tickets would you like {}? ".format(user_name)))
# First, it quantity is not an int, request the user to enter numerical value
# Second, if quantity exceeds remaining tickets, inform customer and ask if they want fewer tickets
# Continue code if neither condition exists
try:
quantity = int(quantity)
if int(quantity) > tickets_remaining:
raise ValueError("There are only {} tickets remaining\nWould you like fewer tickets?".format(tickets_remaining))
except ValueError:
input("Please enter a numerical value: ")
else:
ticket_price = calculate_price(quantity)
confirmation = input("Okay that will cost ${}. Would you like to proceed?\n(Y/N) ".format(ticket_price))
if confirmation.lower() == "y":
print("Thank you {}, your order has been confirmed, please proceed to payment".format(user_name))
tickets_remaining -= int(quantity)
elif confirmation.lower() != "y":
print("Okay then, cya")
else:
print("Okay then, cya")
else:
print("Unfortunately there are no more available tickets, please check back later!")
1 Answer
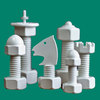
Steven Parker
231,268 PointsHere's a few hints:
- the "except" needs to capture the event object in a variable with an "as" expression
- the event object should be used to create the output message
- without an assignment, the response to an "input" will be lost
- the "except" doesn't need to "input" anyway, the loop will handle that
- a plain "else" will do instead of an "elif" that tests the exact opposite condition from the "if"