Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial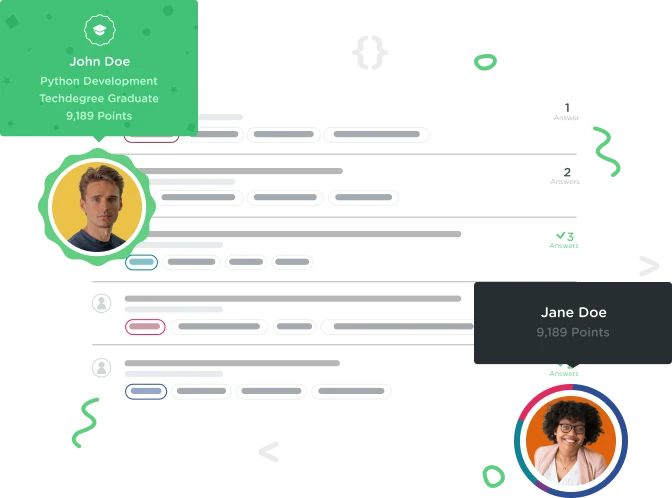

iwgpnjvycw
8,577 PointsHow to handle an array of JSONObjects
Hi everyone! I'm fairly new to Java and Android, and I'm working on an app that retrieves photo data from Unsplash's API. I watched Treehouse's Weather App course, hoping it would give me some idea of how to handle the JSON I'm getting back, but I'm stuck.
I'm getting back an array of JSONObjects, and I'm just not sure of how best to access an object inside an object. For example, each photo object will have a user object, a profile image object, and a urls object.
The JSON looks like this: JSON
What I'm trying to do is display a list of the photos, and each entry will include the photo, the user/photographer's profile image and name, and the number of likes they've gotten for this particular photo.
Below is the code I have so far, but is this even the right way to approach this problem? Is there a way to accomplish this without repeating myself so much?
private NewPhotos getNewPhotos(String jsonData) throws JSONException {
JSONArray unsplash = new JSONArray(jsonData);
for (int i = 0; i < unsplash.length(); i++) {
JSONObject jsonObj = (JSONObject) unsplash.get(i);
String id = jsonObj.getString("id");
String photoUrls = jsonObj.getString("urls");
int likes = jsonObj.getInt("likes");
}
return new NewPhotos();
}
1 Answer
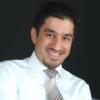
Tareq Alothman
17,921 PointsHello Bianca,
This is my second answer on the forums, and I hope that I can help :)
I think, if you are getting the string "id" correctly, this means you are getting the root of each object correctly. So then, you need to get the objects inside this object to traverse deeper into "user". I think you should use something like the following:
JSONObject jsonObj = (JSONObject) unsplash.get(i);
String id = jsonObj.getString("id");
JSONObject photoUrls = jsonObj.getJSONObject("urls");
String rawUrl = photoUrls.getString("raw");
String fullUrl = photoUrls.getString("full");
...
int likes = jsonObj.getInt("likes");
Good luck :)