Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial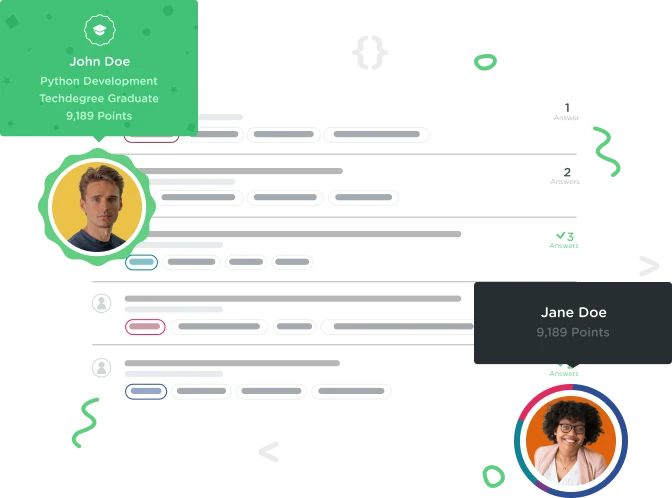
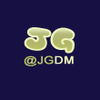
Jonathan Grieve
Treehouse Moderator 91,253 PointsHow to handle importing packages
So I've just worked through a list of 12 compiler error messages to finish this video, which for me is a personal record. ;)
But most of them were needless in that I didn't have the imported packages written in the right order. Below is the order I had them in originally,
import java.util.ArrayList;
import java.io.Serializable;
import java.util.Date;
import java.util.Arrays;
import.java.util.List;
And this is the order I used to get rid of the errors
import java.util.Date;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import java.util.Arrays;
2 questions... why does this matter and how do I know which order to add packages to a problem and get over these errors. I have a feeling IDE's will feature somewhere in the answer :) Thanks
3 Answers

Seth Kroger
56,414 PointsIf you look at your original code I think you'll see a typo on the last line.
I don't think the order itself really matters. The Java compiler will figure it out. IDEs will usually just reorder them alphabetically by groups (java.* and android.* first, then 3rd-party, etc.)
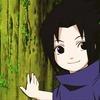
Matthew Francis
6,967 PointsHi Jonathan!i it seems that you are not afar ahed of me. Mind if you share me your code? I seem to lost all my code (not sure why, probably a bug), My code was updated till Sets. But if you also written more code in the Maps video, I don't mind that :) thanks!
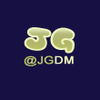
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi Matthew,
I actually got into my workspace today and found my code wasn't there either. Which I thought was odd, but I assumed that was because I downloaded the code to my text editor so ive been working from that for today.
As soon as I power up the laptop again I'll post my code (can't promise it's worth much mind :) )
Or you could contact support, see if there's any chance of getting your code back :). help@teamtreehouse.com
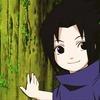
Matthew Francis
6,967 PointsThanks! I guess I'll wait for you, support can take up to 1-3 days haha
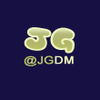
Jonathan Grieve
Treehouse Moderator 91,253 PointsThe latest version of my code so far up. I'm actually a little further now than I was when I posted this thread so I'm up to this video, here. https://teamtreehouse.com/library/java-data-structures/efficiency/menu-ui
Hopefully it won't be long before you get your workspace back
package com.teamtreehouse;
import com.teamtreehouse.model.SongBook;
import com.teamtreehouse.model.Song;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
//new class
public class KaraokeMachine {
//member variables
private SongBook mSongBook;
private BufferedReader mReader;
private Map<String, String> mMenu;
//constructor function for class
public KaraokeMachine(SongBook songBook) {
mSongBook = songBook;
mReader = new BufferedReader(new InputStreamReader(System.in) );
mMenu = HashMap<String, String>();
mMenu.put("add", "Add a new song");
mMenu.put("quit", "Give up, exit the program");
}
//use menu. private to this file. prompt for actions. loop through the menu
private String promptForActions() throws IOException {
System.out.printf("There are %d songs available. Here are your choices? %n", mSongBook.getSongCount() );
//loop through the menu
for(Map.Entry<String, String> option : mMenu.entrySet) {
System.out.printf("%s - %s %n",
option.getKey(),
option.getValue());
}
System.out.print("What do you want to do?");
String choice = mReader.readLine();
return choice.trim().toLowerCase();
}
//build run loop
public void run() {
String choice = "";
do{
try {
choice = promptAction();
switch(choice) {
case "add":
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("%s added! %n%n", song);
break;
case: "quit":
System.out.println("Thanks for playing. See you again")
break;
default:
System.out.printf("Unknown choice. '%s' - Try Again %n%n%n", choice);
}
} catch (IOException ioe) {
System.out.println("Problem with input!");
ioe.printStackTrace();
}
} while(!choice.equals("quit"));
}
}
//prompt for songs method .
private Song promptNewSong() throws IOException {
system.out.print("Enter the artist's name: ");
String artist = mReader.readline();
system.out.print("Enter the title: ");
String title = mReader.readline();
system.out.print("Enter the video URL : ");
String videoUrl = mReader.readline();
return new Song(artist, title, videoURL);
}
}
//import package
package com.teamtreehouse.model;
//new public class
public class Song {
//private unexposed variables - immutable variables. Can only get values not set them.
private String mArtist;
private String mTitle;
private String mVideoUrl;
//use constructor to initiliae variables from member variables.
public Song(String artist, String title, String videoUrl) {
mArtist = artist;
mTitle = title;
mVideoUrl = videoUrl;
}
//expose variables with "getter functions"
public String getTitle() {
return mTitle;
}
public String getArtist() {
return mArtist;
}
public String getVideoUrl() {
return mVideoUrl;
}
//Override Bases toString method -prepare instance to be used as a String.
@Override
public String toString() {
return String.format("Song: %s by %s", mTitle, mArtist);
}
}
package com.teamtreehouse.model;
import java.util.List;
import java.util.ArrayList;
public class SongBook {
//a new private interface List variable.
private List<Song> mSongs;
//constructor SongBook
public SongBook() {
mSongs = new ArrayList<Song>();
}
//public method, returns nothing. takes Song List as a parameter
public void addSong(Song song) {
mSongs.add(song);
}
//
public int getSongCount() {
return mSongs.size();
}
}
//import packages since main file is outside the package.
import com.treamtreehouse.KaraokeMachine;
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
//new public class
public class Karaoke {
//static method named nain that takes array of args returns nothing.
public static void main(String[] args) {
SongBook songBook = new SongBook();
KaraokeMachine machine = new KaraokeMachine(songBook);
//start the loop
machine.run();
}
}
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 Pointsah yes, I see it.
Normally I wouldn't think it'd matter. I've had issues with this before which made me think that there must be some problem with the way the packages were ordered. I guess that dot there must have kept getting in the way without me noticing. :)