Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial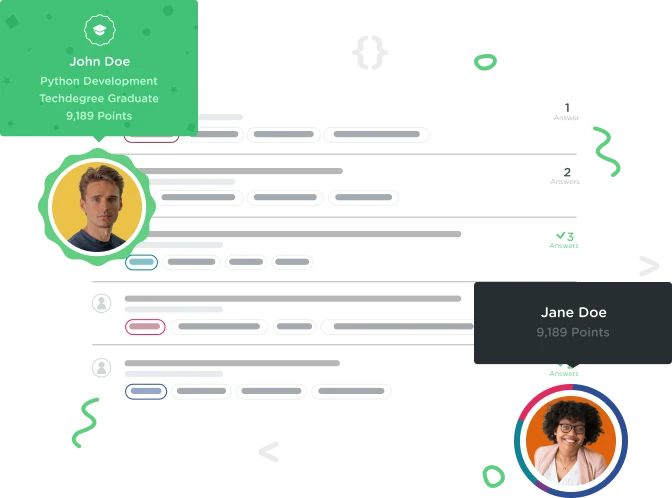

Jacob Keeley
2,033 PointsHow to handle input error?
I recreated the Dungeon Game, following from the videos but implemented a way for the player to choose the size of the square board.
def board():
cells = []
while True:
try:
n = int(input('Specify board size with a single integer:'))
except ValueError:
print("That's not an integer!")
continue
else:
for y in range(1, n+1):
for x in range(1, n+1):
cells.append((x,y))
break
return n, cells
board_size, board_cells = board()
It works well and using the try and except function I've managed to avoid issue with non-integer inputs.
However, this does not handle the error thrown when the player inputs "1", specifying the 1x1 board that would force the monster, player, and door to be on the same square. How can I fix that?
1 Answer

Ken Alger
Treehouse TeacherJacob;
Nice feature. You could certainly use an if
statement to check input to force a minimum board size.
Ken
Jacob Keeley
2,033 PointsJacob Keeley
2,033 PointsThanks, Ken. I thought that'd be the way to go and had been trying to implement it in the try block to no avail. I've since modified the else block, instead.