Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial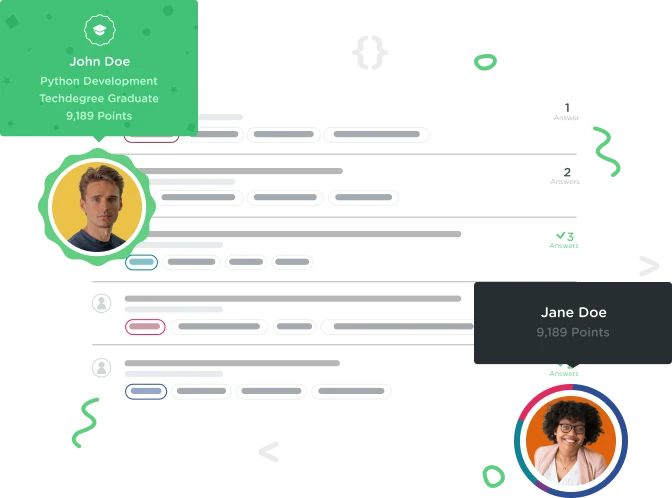
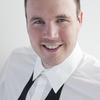
Andrew Reynolds
Front End Web Development Techdegree Graduate 23,333 PointsHow to handle returned objects that have different properties
After retrieving the astronaut objects and trying to add their wiki info to the page, I could only have two out of the 3 people returned load to the page.
Upon checking where the hick-up was, I saw that the object that was not showing up was stuck on the "data.thumbnail.source" part of the generateHTML function. As this Astronaut did not have a thumbnail property.
As well the property for the Astronaut that didn't appear was as follows:
type: "disambiguation"
Has anyone else had this happen?
Below is my code. I had to make a second generateHTML that didn't add an image for the one astronaut that did not have a photo. As well you can see my console.log statements showing where I was checking the Wiki objects for comparisons. Also note the if statement that controls which generateHTML function is called depending on the name of the astronaut.
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
// Make an AJAX request
function getJSON(url, callback) {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload = () => {
if(xhr.status === 200) {
let data = JSON.parse(xhr.responseText);
callback(data);
}
};
xhr.send();
}
// Generate the markup for each profile
function generateHTML(data) {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${data.thumbnail.source}>
<h2>${data.title}</h2>
<p>${data.description}</p>
<p>${data.extract}</p>
`;
}
function generateHTMLNoThumb(data) {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<h2>${data.title}</h2>
<p>${data.description}</p>
<p>${data.extract}</p>
`;
}
btn.addEventListener('click', (e)=>{
getJSON(astrosUrl, (json)=>{
json.people.map(person => {
if (person.name !== 'Andrew Morgan'){
getJSON(wikiUrl + person.name, generateHTML);
} else {
getJSON(wikiUrl + person.name, generateHTMLNoThumb);
}
});
});
// e.target.remove();
});
getJSON(wikiUrl + 'Andrew Morgan', (data)=>console.log(data));
getJSON(wikiUrl + 'Jessica Meir', (data)=>console.log(data));
getJSON(astrosUrl, (data)=>console.log(data));
1 Answer

Chirag Mehta
15,332 PointsHi Andrew, I had the same issue with Andrew Morgan not showing up. The problem is there's more than one Andrew Morgan so the link gets a disambiguation page which doesn't have a thumbnail.
I came across some interesting solutions here.