Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial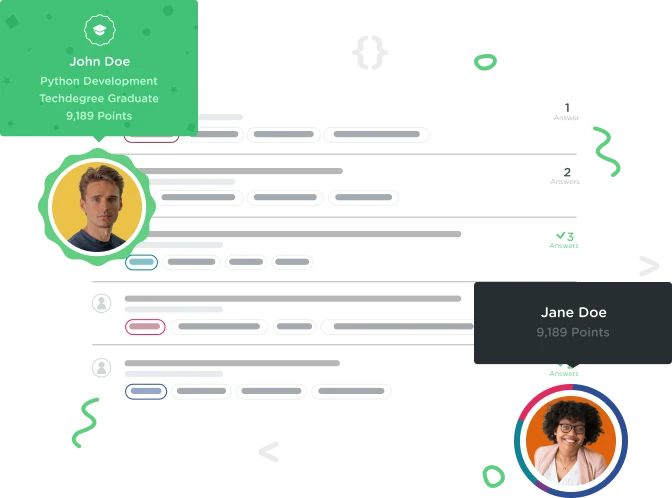
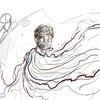
Antonio Rodrigues
1,218 PointsHow to have an input function be able to accept integers and words
Hi, I was wondering if there was a way to have an input function be able to accept words and variables. I'm trying to make a password program but I need the input function to accept both of them. I tried doing this: password = int(input(" create a password ")) but that doesn't accept words. would I need to understand object-oriented programming for this?
1 Answer

Cooper Runstein
11,850 PointsNo, this isn't an issue with objects, it's about understanding types. The problem here is python has no way of converting a string to an interger, and will throw an error if you attempt to do so. One way you could handle this is a try/except block.
password = input("Password: ")
try:
password = int(password)
except ValueError:
password = str(password)
For what you're trying to do though, there isn't any point to converting to an integer. Numbers can be strings, and if a password is entered that isn't fully made of numbers, that program will return a string. For example entering 42Password will return a string. Further you're not considering that the int() function returns integers only, so if you were to enter 5.5, you'd end up with a string '5.5'. If that were then parsed to an integer, you'd have 5. For passwords, the key is to check if two things are the same. It's easier to check if two strings are the same than worry about what format your data is in.
Just remember that all integers can become strings, but not all strings can become integers.
Here's an example of what I'd do instead:
secret = '5.3Secret'
password = input("password: ")
if str(password) == secret:
print("Welcome")
Antonio Rodrigues
1,218 PointsAntonio Rodrigues
1,218 PointsThank you that helped alot