Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial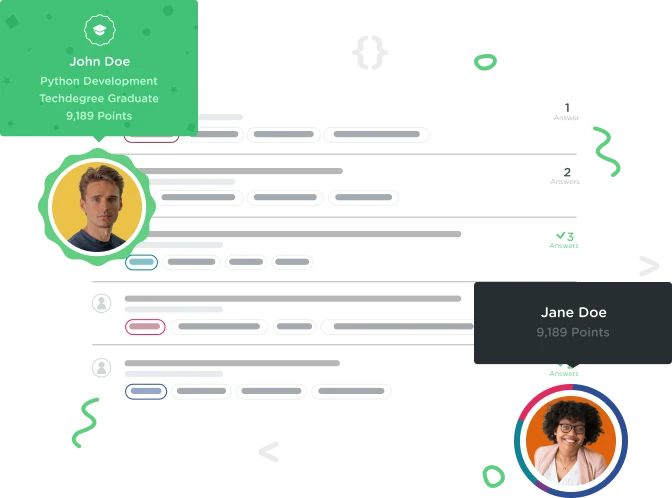
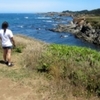
Nancy Melucci
Courses Plus Student 36,158 PointsHow to implement collect method in functional programming challenge
Since this challenge does not seem to require that we create a Stream method, I am unsure how to implement the .collect method in a manner similar to that in the video...my code which runs but does not pass two of the tests, follows...
package com.teamtreehouse.challenges.highscores;
import com.teamtreehouse.challenges.highscores.model.Score;
import com.teamtreehouse.challenges.highscores.service.ScoreService;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
ScoreService service = new ScoreService();
List<Score> scores = service.getAllScores();
System.out.printf("There are %d total scores. %n %n", scores.size());
System.out.println("Imperatively - First 3 'Nintendo Entertainment System' Scores");
List<Score> nintendoScores = getFirstThreeNintendoScoresImperatively(scores);
nintendoScores.forEach(System.out::println);
System.out.println("Declaratively - First 3 'Nintendo Entertainment System' Scores");
// NOTE: Reassigning here
nintendoScores = getFirstThreeNintendoScoresDeclaratively(scores);
nintendoScores.forEach(System.out::println);
}
public static List<Score> getFirstThreeNintendoScoresImperatively(List<Score> scores) {
List<Score> result = new ArrayList<>();
// Four score and 7 years ago....#dadjoke
for (Score score : scores) {
if (score.getPlatform().equals("Nintendo Entertainment System")) {
result.add(score);
if (result.size() >= 3) {
break;
}
}
}
return result;
}
public static List<Score> getFirstThreeNintendoScoresDeclaratively(List<Score> scores) {
// TODO: Filter the scores stream to be of platform 'Nintendo Entertainment System'
List<Score> nintendoScores = new ArrayList<>();
for (Score score : scores) {
String platform = score.getPlatform().toLowerCase();
if (platform.contains("Nintendo")) {
nintendoScores.add(score);
if (nintendoScores.size() > 3){
break;
}
}
}
// TODO: Limit it to 3 scores
// TODO: Return a newly collected list using the collect method
return nintendoScores;
}
public static void printBurgerTimeScoresImperatively(List<Score> scores) {
for (Score score : scores) {
if (score.getGame().equals("Burger Time") && score.getAmount() >= 20000) {
System.out.println(score);
}
}
}
public static void printBurgerTimeScoresDeclaratively(List<Score> scores) {
scores.stream()
.filter(score -> score.getGame().equals("Burger Time"))
.filter(score -> score.getAmount() >= 20000)
.forEach(System.out::println);
}
}