Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial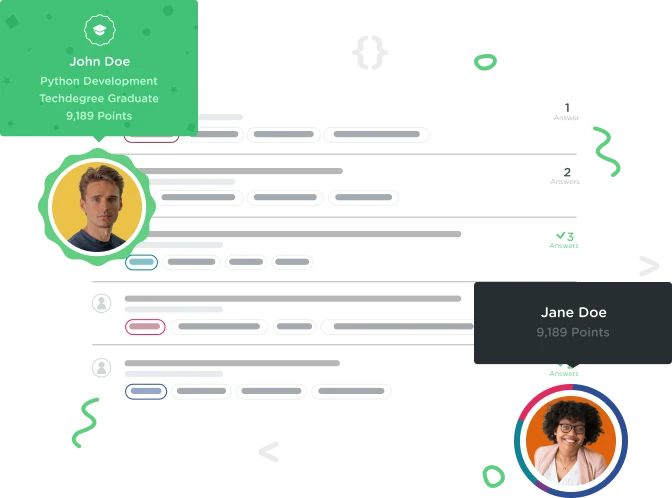

Rohit Thorat
12,191 Pointshow to implement nextId in this code
Application.js
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import Header from './Header';
import Player from './Player';
import AddPlayerForm from './AddPlayerForm';
import './App.css';
class Application extends Component {
constructor(props){
super(props);
this.state = {
playerState: this.props.initialPlayers
};
}
onScoreChangeApp(index,delta) {
// console.log("onScoreChangeApp",index,delta);
this.state.playerState[index].score += delta;
this.setState(this.state);
}
onPlayerAdd(name) {
console.log("name added",name);
this.state.playerState.push({
name: name,
score: 0,
id: 4,
});
this.setState(this.state);
}
render(){
return(
<div className="scoreboard">
<Header titleHeader={this.props.titleApp} playerHead={this.props.initialPlayers}/>
<div className="players">
{this.state.playerState.map(function(p,index) {
return (
<Player onScoreChange={function(delta) {this.onScoreChangeApp(index,delta)}.bind(this)}
name={p.name}
scoreP={p.score}
key={p.id} />
);
}.bind(this))}
</div>
<AddPlayerForm onAdd={this.onPlayerAdd.bind(this)}/>
</div>
);
}
}
Application.propTypes = {
titleApp: PropTypes.string,
initialPlayers: PropTypes.arrayOf(
PropTypes.shape({
name: PropTypes.string.isRequired,
score: PropTypes.number.isRequired,
id: PropTypes.number.isRequired
})).isRequired
};
Application.defaultProps = {
titleApp: "Scoreboard",
};
export default Application;

Rohit Thorat
12,191 Pointsi am pushing a new player in the array using onPlayerAdd method . Here my id value is hard coded i.e.
this.state.playerState.push({
name: name,
score: 0,
id: 4,
});
i want to use
var nextId = 4
and replace it in the code like
id: nextId
and then increase nextId by 1 , i tried using this -
onPlayerAdd(name) {
var nextId = 4;
console.log("name added",name);
this.state.playerState.push({
name: name,
score: 0,
id: nextId,
});
this.setState(this.state);
nextId += 1;
}
but it is not increasing the value of nextId
2 Answers

Tim Renner
11,163 PointsRohit, try adding a state variable nextId in your component Constructor, right below playerState. Give it an initial value.
Then use it and increment it in your onPlayerAdd function:
this.state.playerState.push({
name: name,
score: 0,
id: this.state.nextId,
});
this.setState((prevState) => {
return {nextId: prevState.nextId++};
});

Mike Jensen
11,718 PointsYou might consider using shortid. It's an npm library which "creates amazingly short non-sequential url-friendly unique ids".
You just need to:
- install it:
npm install shortid
- import it:
import shortid from 'shortid
- use it:
id: shortid.generate()
Rohit Thorat
12,191 PointsRohit Thorat
12,191 Pointsindex.js