Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial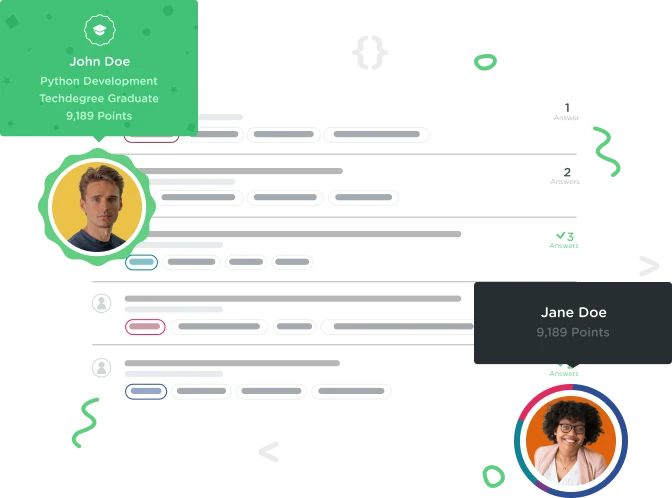

Christiano Palmezan
Courses Plus Student 3,289 PointsHow to implement the session logic based on the "role" of the user? I want to show some contents
I'm trying to show some menu itens for the admin and hide for those whose aren't admin.
Just that!
Tks
1 Answer
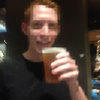
Sebastian H
19,905 PointsI imagine you'd take a very similar process to how userId was made available to jade/pug in the video. I'm really not coming from an experienced enough standpoint to say this is the right way BUT just for the sake of potentially helping i'd just follow the same process Dave did with the userId:
1 - You'll need to add a user role to your user Schema. For my example i've just gone with an admin property with a default of false. This means that later on in jade/pug you will just be able to eval if the admin property === true
var UserSchema = new Schema({
f_name: {
type: String,
required: true,
trim: true
},
admin: {
type: Boolean,
default: false
}
});
2 - I'm not sure if it would be best to
a. Add this new user.admin to a cookie with the userId. I'm tempted to do this because we have already queried the database in the authenticate method and our specific user is found and readily available. There may be security concerns with storing user role in a cookie though, I have no idea.
b. alternatively, it might be better to add a new middleware function which again query's for a specific user doc, this will keep the user.admin role out of a cookie.
Assuming it's ok to add user.admin to a cookie (option a) you would edit your /login post route to store user.admin in the req.session object.
router.post('/login', function(req, res, next) {
if(req.body.email && req.body.password) {
User.authenticate(req.body.email, req.body.password, function(error, user) {
if(error || !user ) {
var err = new Error('Wrong email or password.');
err.Status = 401;
return next(err);
} else {
req.session.userId = user._id;
// added this new line which stores user role in cookie
req.session.userIsAdmin = user.admin;
return res.redirect('http://localhost:3000/');
}
});
} else {
var err = new Error('All fields are required.');
err.Status = 401;
return next(err);
}
});
3 - Now we make the user role available to jade/pug. Do this by adding it to the res.locals object. (main app.js file):
// make userId and userIsAdmin available to jade/pug
app.use(function(req, res, next) {
res.locals.currentUser = req.session.userId;
res.locals.userIsAdmin = req.session.userIsAdmin;
next();
});
4 - Finally, you have access to the user role in jade/pug.
// check if userIsAdmin === true
if userIsAdmin
// show special admin menu buttons