Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial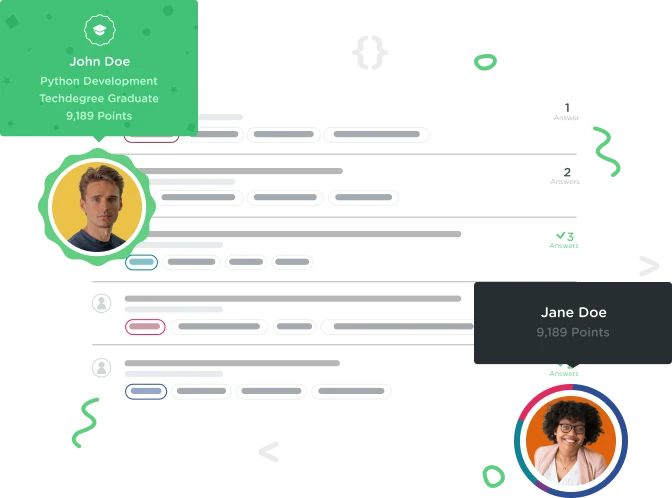
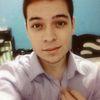
sammarxz
Front End Web Development Techdegree Student 7,815 PointsHow to implement this in code?
If the user places over a number display this way:
number 1: 0800 - number 2: 9090
if not, you will usually show:
number: 9090
how the logic ?
6 Answers
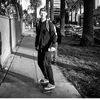
Joshua Paulson
37,085 PointsCould you please revise the question? I don't really understand what you're asking.
Would you like to add an additional number to the contact list phone number?
You might need to add the Dash ( - ) between sets of numbers.

David O' Rojo
11,051 PointsIt is an easy task if you rely on the number of phones that each contact has to choose how to print them. If the number of phones is one, you print them the normal way, but there are more than one number, you print them numbering their occurrence.
The snippet below illustrates the logic:
def print_phones(list)
if list.length > 1
list.length.times do |index|
puts "Phone #{index + 1}: #{list[index]}"
end
else
list.each do |phone|
puts "Phone: #{phone}"
end
end
end
some_phones_1 = ['1234', '5678']
print_phones some_phones_1
# => Phone 1: 1234
# => Phone 2: 5678
some_phones_2 = ['8000']
print_phones some_phones_2
# => Phone: 1234
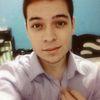
sammarxz
Front End Web Development Techdegree Student 7,815 PointsExemple:
What is the person's name? Sam
Do you want to add a phone number? (y/n) y
Enter a phone number: 9090
Do you want to add a phone number? (y/n) y
Enter a phone number: 0800
Do you want to add a phone number? (y/n) n
Add another? (y/n) n
----
Name: Sam
Phone 1: 9090
Phone 2: 0800
----
you got it ? Place the numbering side, to identify the telephone number.
- phone 1,
- phone 2
- phone 3 ...
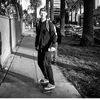
Joshua Paulson
37,085 PointsSorry, Still not really understanding what you're trying to do. What do you mean by numbering side? Are you trying to implement the phone type? Or are you trying to combine 0800 and 9090 into 0800-9090?
Please be very specific
phone-number.rb
class PhoneNumber attr_accessor :kind, :number
def to_s "#{kind}: #{number}" end end
address-book.rb
when 'p' phone = PhoneNumber.new print "Phone number kind (Home, work, etc):" phone.kind = gets.chomp print "Number: " phone.number = gets.chomp contact.phone_numbers.push(phone)
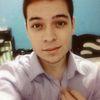
sammarxz
Front End Web Development Techdegree Student 7,815 Pointsin, if you have more than one number, I would like to arrange and place the numbering
as the phone number and another phone
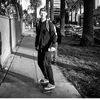
Joshua Paulson
37,085 PointsAlright I think I've got it. You'd like to show both phone numbers on a single line? Name: # Phone Numbers: # and #
If that's what you're trying to do then you'd have to change the syntax so the conditional statement takes two arguments instead of only one.
The code you have is set for one number at a time.
phone = PhoneNumber.new
print "Phone number kind (Home, work, etc):"
phone.kind = gets.chomp
print "Number: " < THIS is part of code you'd need to modify so that it prints all numbers instead of one >
phone.number = gets.chomp
contact.phone_numbers.push(phone)
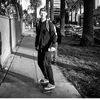
Joshua Paulson
37,085 PointsI'll come back to your question very soon. I'm going to link up with a good friend of mine from Brazil and see if he can help us out.
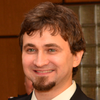
Miroslav Kovac
11,454 PointsHere is my function with each_with_index
def print_contact_list(contact_list)
puts "-" * 50
contact_list.each do |contact|
puts "*" * 50
puts "Name: #{contact[:name]}"
contact[:phones].each_with_index do |phone, index|
puts "Phone ##{index + 1}: #{phone}"
end
puts "*" * 50
end
puts "-" * 50
end
Output:
Name: Jake Phone #1: 123456 Phone #2: 456789
Name: John Phone #1: 89756