Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial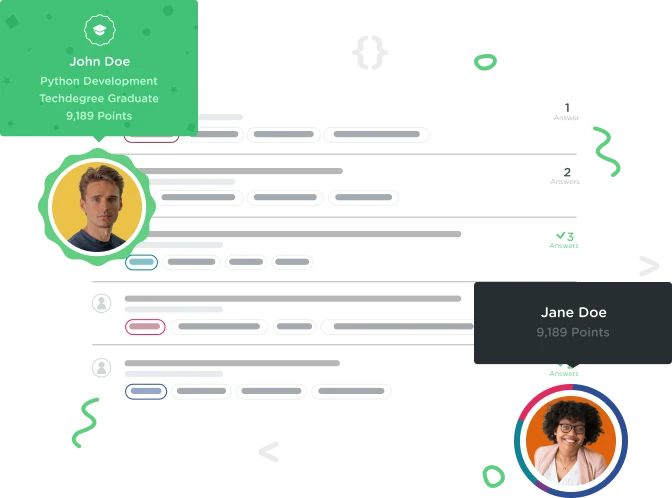
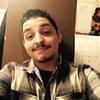
Jose Medina
Front End Web Development Techdegree Graduate 15,656 PointsHow to improve this code?
//My code
var quizLines = [
['What is this browser?', 'chrome'],
['What is the color of water?', 'blue'],
['What is the color of the sky?', 'blue']
];
var quizRight = [];
var quizWrong =[];
var questionsR;
var numCorrect = 0;
var html;
var rightMessage;
//Display messages
function print(message) {
document.write(message);
};
function printR() {
document.write('<p>You got these correct.</p>');
document.write('<p>'+ quizRight.join('<p> ') + '</p>');
};
function printW() {
document.write('<p>You got these wrong.</p>');
document.write('<p>'+ quizWrong.join('<p> ') + '</p>');
};
// Loop
for (var i = 0; i <=2 ; i += 1) {
//Question loop
var sAnswer = prompt( quizLines[i][0] );
sAnswer = sAnswer.toLowerCase();
var correctA = quizLines[i][1];
//Correct counter
if (sAnswer === correctA) {
numCorrect += 1;
quizRight.push(quizLines[i][0]);
} else {
quizWrong.push(quizLines[i][0]);
}
};
html = "<p>You got " + numCorrect + " question(s) right.</p>"
print(html);
printR();
printW();
It works more or less but I feel like it could be improved.
1 Answer

Stephan Olsen
6,650 PointsThere are several ways you can improve the code. I don't know how much JavaScript you know, so I wont name everything that comes to mind. One that I think is quite useful for your code, is to iterate your loop depending on the amounts of questions in your array, instead of a static number. Otherwise if you forget to update the for loop, you will suddenly miss a question.
for (var i = 0; i < quizLines.length ; i += 1) {
I also see that you're declaring a variable rightMessage which you don't seem to be using anywhere. Delete it if you're not going to use it. Also there's no real reason to declare your html variable when you're not using it until the end. You should just initialize there with the value. It makes more clean code, especially when the code gets longer and more complex. I would definetely also create the quizLines as an array of objects, rather than an array of arrays. This would make your code cleaner and more readable, as you could give the properties names instead of using their index.