Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial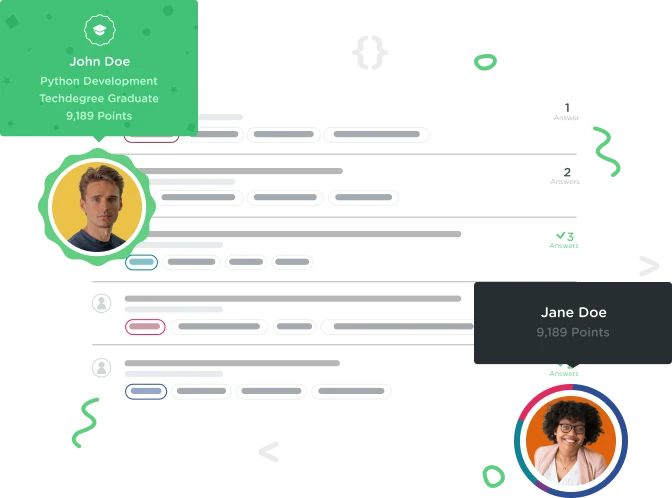

Zeyad Salah Elhennawi
1,586 Pointshow to initialise instances into an inherited class?
If I have a class and make a new one which is an inherited one and added some instance. should I initialise its constants and the inherited ones?
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int
init (numberOfSeats: Int){
self.numberOfSeats = numberOfSeats
}
}
let someCar = Car(numberOfSeats: 4)
1 Answer
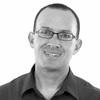
David Papandrew
8,386 PointsLazy way to do it (what I would do):
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int = 4
}
let someCar = Car(withDoors: 4, andWheels: 4)
Since you set a default value for numberOfSeats, no need to create new initializer. New instance of this subclass will use the default property and all you have to do is create new instance with the parent class initializer.
If you must create a new initializer, this is how it would look:
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int = 4
init(withDoors doors: Int, andWheels wheels: Int, numberOfSeats: Int) {
self.numberOfSeats = numberOfSeats
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 4, andWheels: 4, numberOfSeats: 4)