Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial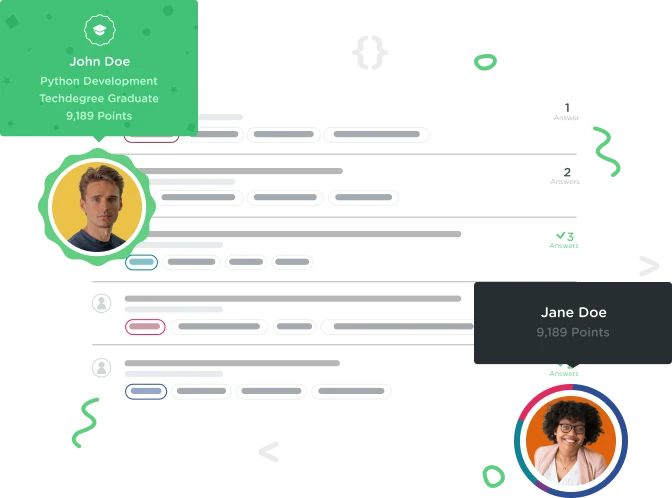
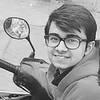
Kanish Chhabra
2,151 PointsHow to initialize additional values of the subclass within subclass
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car: Vehicle {
var numberOfSeats: Int = 4
override init (withDoors doors: Int, andWheels wheels: Int, numberOfSeats: Int)
{
self.numberOfSeats = numberOfSeats
super.init(withDoors: doors, andWheels: wheels)
}
}
How do I initialize "numberOfSeats" within subclass "Car"
[MOD: edited code block - srh]
5 Answers
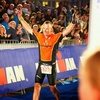
Steve Hunter
57,712 PointsAlso, as the init
method here is identical to the superclass, does it need to be there? The call to super init
is implied, I think. So, does the new subclass just need to look like:
class Car : Vehicle {
var numberOfSeats: Int = 4
// omit the rest?
// override init (withDoors doors: Int, andWheels wheels: Int) {
// super.init(withDoors: doors, andWheels: wheels)
// }
}
Just thinking aloud but it's worth a try!
(I'll try it in the challenge ... )
[EDIT]
Yep - this worked in the challenge - I've not tried it in Xcode:
class Car : Vehicle {
var numberOfSeats: Int = 4
}
let someCar = Car(withDoors: 4, andWheels: 4)
[EDIT2]
Yes, this also works in Xcode so the additional init
method isn't required as it adds nothing to the super init
method that is already defined. There's no override
as the parameters being passed in are identical. Creating a subclass automatically calls the superclass init
method. The new member variable, numberOfSeats
is set within the Car
class as a default value so doesn't need to be set by the subclass init
method.
Make sense?
Steve.
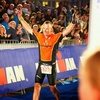
Steve Hunter
57,712 PointsHI there,
You've done it already! But take the mention of numberOfSeats
out of the init
method. Just declare the numberOfSeats
variable, call it an Int
and assign the value 4 to it at the top of the class; just like you have done. And then, that's it!
class Car : Vehicle {
var numberOfSeats: Int = 4
override init (withDoors doors: Int, andWheels wheels: Int) {
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 4, andWheels: 4)
I hope that helps.
Steve.
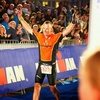
Steve Hunter
57,712 PointsHi Kanish,
This works:
class Car : Vehicle {
var numberOfSeats: Int
init(withDoors doors: Int, andWheels wheels: Int, andSeats seats: Int){
self.numberOfSeats = seats
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 4, andWheels: 4, andSeats: 7)
Let me know if you want me to walk you through that code - I think you'll get it just fine.
Steve.
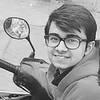
Kanish Chhabra
2,151 PointsSure. I did it your way, omitted the unnecessary 'override initializer' and I added the normal initializer for initialising value to 'numberOfSeats'. It worked but I wasn't able to initialize the value to 'numberOfDoors' and 'numberOfWheels'

Emin Grbo
13,092 PointsYou rock Steve !! :)) This totally makes sense. I was a bit confused why are we re-writing init method if it is already there. Now it is much clearer :))
Sometimes exercises get a bit confusing and make me thing something HAS TO BE DONE in that way. But i see it is better to experiment a bit and just make something that works ;)
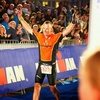
Steve Hunter
57,712 PointsHey, no problem! Glad to help.
Kanish Chhabra
2,151 PointsKanish Chhabra
2,151 PointsYeah I got it, but what if I wanted to initialize numberOfSeats using init method within the subclass?
Kanish Chhabra
2,151 PointsKanish Chhabra
2,151 PointsBtw, Thanks a lot :)
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHiya,
I'm out at the moment but I'll fire up Xcode when I get home and get an answer over to you ASAP.
I don't think you were too far off with your first suggestion.
Steve.