Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial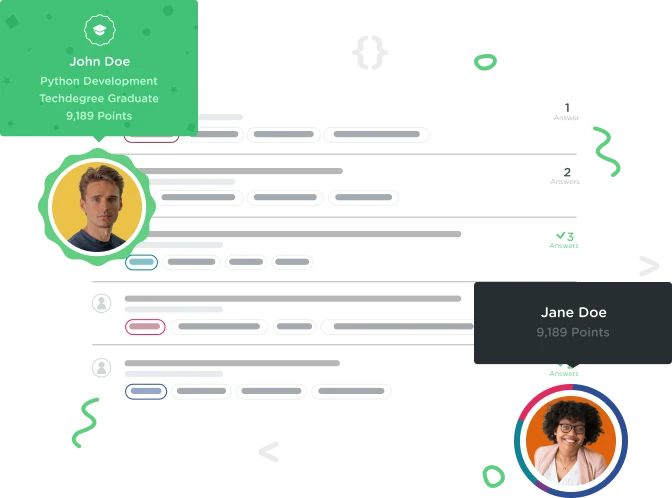

lvwdhydynw
6,443 PointsHow to initialize an array in initializer without giving it any value?
Alright, so - this is my code:
struct Club {
var name: String
var country: String
var squad: [Player]?
var captain: Player?
init(name: String, country: String) {
self.name = name
self.country = country
self.captain = nil
self.squad = nil
}
}
struct League {
var name: String
var country: String
var currentChampion: Club?
var teams: [Club] = []
init(name: String, country: String) {
self.name = name
self.country = country
currentChampion = nil
teams.append(Club(name: "A", country: "A"))
teams.removeAll()
}
}
There's also a structure "Player", but that's not important right now so I didn't include it. Everything important is in structure League. I have declared variable "teams" which is an array consisting of instances of struct Club. And now - I want to initialize it without giving it any (default) value as it wouldn't make any sense here. But so far, to do so, this is the only way I have found which works - that in init method I add a completely random club to the array teams and on the next line I remove it.
Isn't there a better way to do so? I really can't think of any.
PS: Above, in structure Club, you may see that I tried to use optionals and that worked till I actually tried to append an instance of club to the array when it simply said this array is still empty
1 Answer

lvwdhydynw
6,443 PointsJeff McDivitt I probably should have said that I've just learned about structures and thus I want to practise them. It's not any real project, it's just for learning about structures, their limitations etc :)
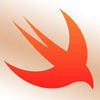
Jeff McDivitt
23,970 PointsGot it - Yeah that would be awful tough with structs, but as you progress you will find easier ways to do things.
Jeff McDivitt
23,970 PointsJeff McDivitt
23,970 PointsWhy are you using structures for this; you could easily do what you need using protocols.