Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial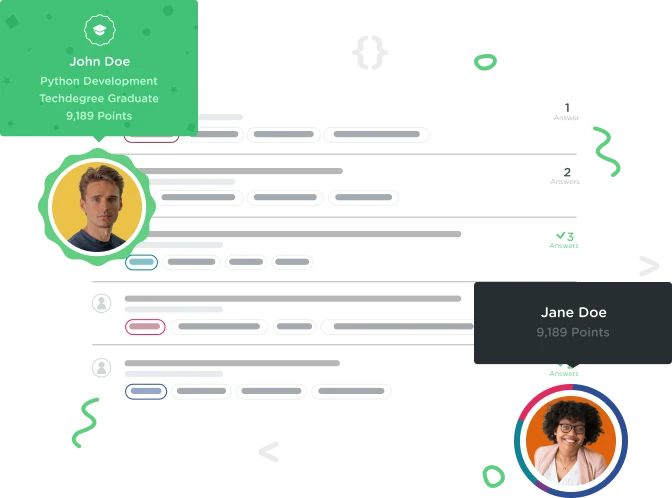

Zachary Vacek
11,133 PointsHow to input and return a list of dates and links?
I've figured out how to return an enumerated list of links after the user inputs some dates. Here is my code:
import datetime
date_list = []
answer_format = "%m/%d"
link_format = "%b_%d"
link = "https://en.wikipedia.org/wiki/{}"
while True:
print("What date would you like? Please use MM/DD format. Enter 'quit' to quit. Use 'done' to print links. ")
answer = input("> ")
if answer.upper() == "QUIT":
break
elif answer.upper() == "DONE":
for index, item in enumerate(date_list, 1):
print("{}: {}".format(index, item))
try:
date = datetime.datetime.strptime(answer, answer_format)
output = link.format(date.strftime(link_format))
date_list.append(output)
except ValueError:
print("That's not a valid date. Please try again.")
But what I really want to do is have the program only ask for user input once, and the user can input a list of dates, seperated by commas, like this: "01/01, 02/02, 03/03..."
Then the program would then return a list of links.
Is that possible, and if so, can anyone give me a hint as to how to make that work?
Thanks! Zac
2 Answers
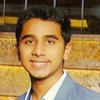
Anish Walawalkar
8,534 PointsThis is how I would do it:
import datetime
import sys
date_list = []
answer_format = "%m/%d"
link_format = "%b_%d"
link = "https://en.wikipedia.org/wiki/{}"
def create_wiki_time_links(dates):
for date in dates:
try:
date_format = datetime.datetime.strptime(date, answer_format)
output = link.format(date_format.strftime(link_format))
date_list.append(output)
except ValueError:
print('{} is not a valid date'.format(date))
def get_dates_from_user():
print("What date would you like? Please use MM/DD format. Enter 'quit' to quit. Use 'done' to print links. ")
answer = raw_input("> ")
dates = answer.split(', ')
create_wiki_time_links(dates)
def print_dates():
for index, link in enumerate(date_list):
print('{}: {}'.format(index, link))
def main():
get_dates_from_user()
print_dates()
if __name__ == '__main__':
try:
main()
except KeyboardInterrupt:
sys.exit()
Since you only want to run the module once, I would suggest not running it in a while true loop. Also since you want the user to input all the dates on the same line separated by commas (;) you should call the split(', ') method on the answer. Check out my code. I'm using raw_input instead of input as I coded it with python 2.7

Jason Whitaker
12,252 Pointsimport datetime
import sys
date_list = []
answer_format = "%m/%d"
link_format = "%b_%d"
link = "https://en.wikipedia.org/wiki/{}"
def create_wiki_time_links(dates):
for date in dates:
try:
date_format = datetime.datetime.strptime(date, answer_format)
output = link.format(date_format.strftime(link_format))
date_list.append(output)
except ValueError:
print('{} is not a valid date'.format(date))
def get_dates_from_user():
print("What dates would you like? Use the MM/DD format. Enter 'quit' to quit. Use 'done' to print links")
answer = input("Dates: ")
dates = answer.split(', ')
create_wiki_time_links(dates)
def print_dates():
for index, link in enumerate(date_list):
print('{}: {}'.format(index, link))
def main():
get_dates_from_user()
print_dates()
if __name__ == '__main__':
try:
main()
except KeyboardInterrupt:
sys.exit()
Modified to work on Python 3
Zachary Vacek
11,133 PointsZachary Vacek
11,133 PointsAwesome, thanks for the help Anish! Very helpful indeed.