Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial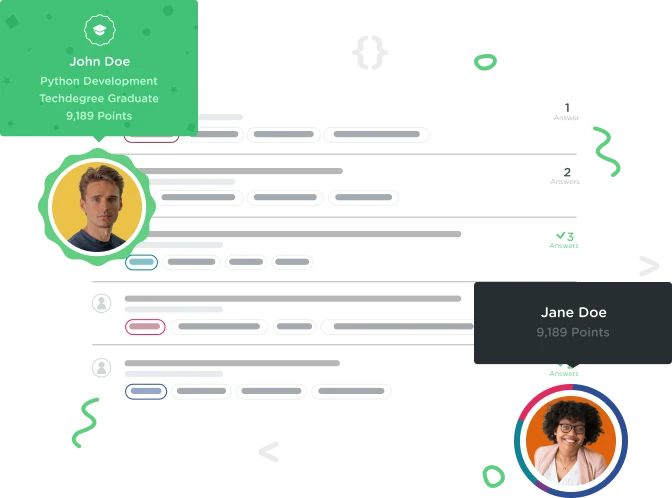

Gia Hoang Nguyen
4,137 PointsHow to input tips?
import math
def split_check(total, number_of_people,tips):
cost_per_person = math.ceil(total / number_of_people)
return cost_per_person
total_due = float(input("What is the total? "))
number_of_people = int(input("How many people? "))
amount_due = split_check(total_due,number_of_people)
print("Each person owes ${}".format(amount_due))
If I want to create another feature like tips in percentage? what should I do?
MOD: I just updated your code with the markdown that will make it a lot easier for others to read. See the link to the markdown cheatsheet just below the text box for how to do this. It's easy! :-) Thanks!
1 Answer
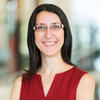
Louise St. Germain
19,424 PointsHi Gia,
If you wanted to factor in a tip where you give it a percentage, you would want to update the total before you did the division that gave you cost per person. In other words, something like this:
import math
def split_check(total, number_of_people, tip_percentage):
# New line of code here: total = total + a percentage of the total as a tip
total += total*tip_percentage # Would need to be a decimal, like 0.15 is 15%
# The rest of the code stays the same
cost_per_person = math.ceil(total / number_of_people)
return cost_per_person
total_due = float(input("What is the total? "))
number_of_people = int(input("How many people? "))
# Need to ask for a tip percentage:
# TODO: Add the code here - you know how to do this.
# Notice there is now one more parameter to split_check, for the tip.
amount_due = split_check(total_due,number_of_people, tip_percentage)
print("Each person owes ${}".format(amount_due))
Hopefully it's not too complicated! Let me know if you still have questions and I'm happy to help.
thomas shepard
278 Pointsthomas shepard
278 PointsI can't figure out how to input the answer to "What is the total?" I am working with Idle as well as in the console of Sublime.
Louise St. Germain
19,424 PointsLouise St. Germain
19,424 PointsHi Thomas,
I have Sublime also, but I generally have an external console open to run the program. (So I have 2 windows open: one for editing the program with Sublime text, and a separate command prompt window for running it.) In my case I'm on Windows so I run the program in the Command Prompt (PowerShell works too) by navigating to the directory where my program is, and typing "python [my_program_name].py" at the prompt. On a Mac the terminal window does the same thing.
When I'm in Sublime and I just hit Ctrl+B to build out the program within Sublime, I get the right output if it's just printing things to the console, but it doesn't work properly if it needs input, i.e it will print the question but there's no way to answer.
Without knowing more about your set up it's hard to tell if this is the same problem you're experiencing. If you try running the program from outside Sublime using a command prompt (Windows) or terminal (Mac), does it work?