Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial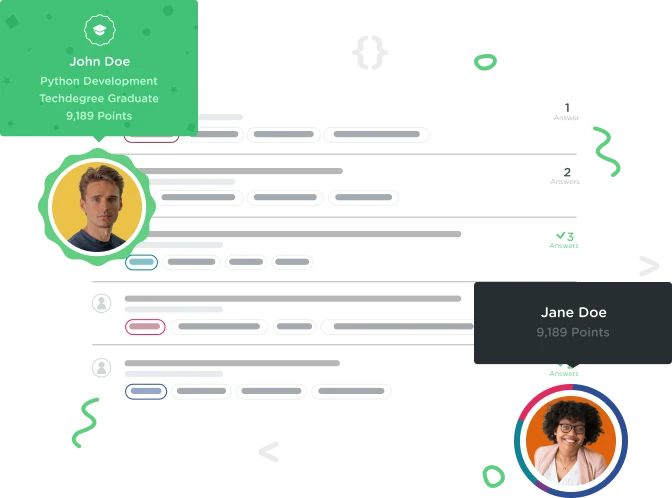

Mayur Pande
Courses Plus Student 11,711 PointsHow to integrate pdo with slim
How would this work with slim routing?
2 Answers
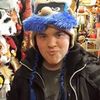
Codin - Codesmite
8,600 Points<?php
function db() {
static $db = null;
if (null === $db)
$db = new PDO(...);
return $db;
}
?>
:)
Your PDO connection details inplace of "..."
I would also reccomend storing your connection details outside of your websites root folder inside a config file for extra security.
Good explination here using sqli, but can easily be adapted using the same method for PDO: https://www.binpress.com/tutorial/using-php-with-mysql-the-right-way/17

Mayur Pande
Courses Plus Student 11,711 PointsAshley Skilton thanks anyway, managed to get it sorted doing it without slim. I ended up asking at stack even there someone was having difficulty helping me! I agree with you as well their community can be very blunt sometimes.
Mayur Pande
Courses Plus Student 11,711 PointsMayur Pande
Courses Plus Student 11,711 PointsHi, thanks for the answer, but I am still confused how to set up routing with the slim framework. I get how to make the connection to the database and retrieve results. For example I have a portfolio page where the url is /portfolio and I have items on there that I want to link to another template. So when I click a link item it will take me to another page (template using twig) that would have the url /portfolio/id where the id is obtained from mysql. I have done this so far using procedural code ;
This is my index.php file
This is my main.twig file;
This is my porfolio.twig file;
and finally this is my portfolioitem.twig file;
However when I run these it seems not to work when I click one of the links. However if I manually put the id number in the browser (/portfolio/1) it renders the porfolio.twig file however my stylesheet is not applied to it. I have no idea where I have gone wrong.
Codin - Codesmite
8,600 PointsCodin - Codesmite
8,600 PointsAh, I unfotunately do not have enough experience with slim to answer this. Hopefully another member has a solution.
If not it is well worth asking at www.stackoverflow.com, there is a community of very knowledgable programmers over there, I find the community a little more blunt and maybe less friendly, but you are sure to get a good answer if you tag your question with the relevant tags (PHP, Slim, PDO) etc
If you do get a good answer definately post it here so others can benifet from the answer :)