Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial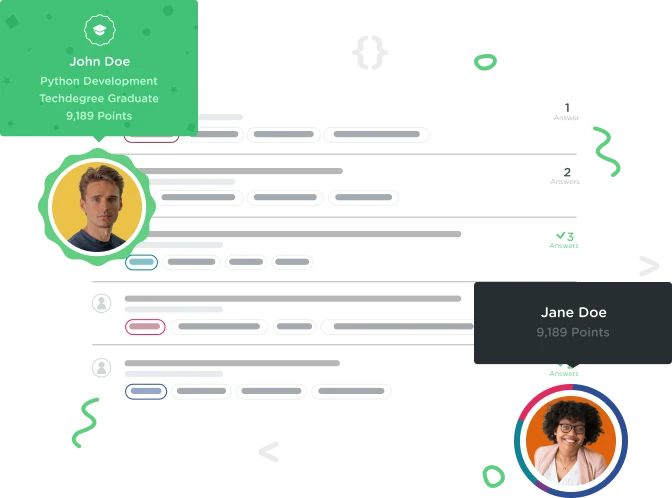

Wagner Neves
Courses Plus Student 501 PointsHow to iterate through a list, turning every value into integers, and using this "new" integer within a FOR loop?
Hi. I was practicing with the workshop Basic Math Calc in Python. The exercise asks us to:
Step 1
Ask the user for their name and the year they were born.
Step 2
Calculate and print the year they'll turn 25, 50, 75, and 100.
Step 3
If they're already past any of these ages, skip them.
I came up with the solution below:
userName = input("What is your name? ") bornYear = int(input("What year were you born? ")) currentYear = 2017 userAge = currentYear - bornYear ages = [25, 50, 75, 100]
for turnAge in ages: ((ages - userAge)+2017) if ages < userAge: continue print("Hi {}. You are {} years old. You will complete {} in the year {}".format(userName, userAge, ages, turnAge))
The code asks my name and the year I was born, but when it gets to the loop part, it comes back with this error:
"Traceback (most recent call last):
File "age_calc.py", line 17, in <module>
((ages - userAge)+2017)
TypeError: unsupported operand type(s) for -: 'list' and 'int'"
I understand a list is a list, so I need firstly to turn every element inside the list into an integer. How can I do that using a FOR loop, like I was trying to do?
6 Answers

Damien Watson
27,419 PointsHi, you are already looping through the 'ages' list, which has the ages stored as numbers, you're just not using them in the correct way. You are also doing a calculation with no purpose, so I have commented it out.
Set up variables
userName = input("What is your name? ")
bornYear = int(input("What year were you born? "))
currentYear = 2017
userAge = currentYear - bornYear
ages = [25, 50, 75, 100]
Calculation
for turnAge in ages:
# turnAge will loop through 25, 50, 75 & 100
# not needed -> ((ages - userAge)+2017)
if currentYear > (bornYear + turnAge):
continue
print("Hi {}. You are {} years old. You will complete {} in the year {}".format(userName, userAge, turnAge, bornYear +turnAge))

Nathan Burnham
7,827 PointsCounting from 0 you can access a number in a list ages[0] is 25, for example.
You'd want to set it up like so: for age in len(range(ages)): print(ages[age])
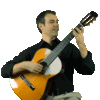
Stephen Hanlon
11,845 Pointsdef future_ages():
# Name
name = input("What's your name? ")
# Age
while True:
year_born = input("What year were you born? ")
try:
year_born = int(year_born)
except ValueError:
continue
else:
break
# The Facts
current_year = 2018
now_age = current_year - int(year_born)
ages = [25, 50, 75, 100]
# Holy moly! Am I really that old!!
for age in ages:
if now_age < age:
future_age_year = (age - now_age) + current_year
response = "{}, you'll be {} in {}.".format(name, age, future_age_year)
print(response)
else:
continue

Istvan Nonn
2,092 Pointsimport datetime
now = datetime.datetime.now()
year = now.year
# Step 1
# Ask the user for their name and the year they were born.
name = input("Please enter your name: ")
while True:
year_of_birth = input("Hey {}, now please enter your birth year: ".format(name))
try:
year_of_birth = int(year_of_birth)
except ValueError:
print("Ok, please enter a 4 digit number for birth year!")
continue
else:
print("Thank you!")
break
# Step 2
# Calculate and print the year they'll turn 25, 50, 75, and 100.
anniversaries = [25, 50, 75, 100]
anniversary_year = []
for celebration in anniversaries:
anniversary_year.append(year_of_birth + celebration)
# Step 3
# If they're already past any of these ages, skip them.
k = 0
for party in anniversary_year:
if party > year:
print("{}, it looks like your {} anniversary will be in {}".format(name, anniversaries[k], party))
k += 1
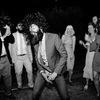
Ian Vidaurre
3,931 PointsMy answer was a bit messier but I was able to make it work. Next step will be cleaning and refactoring!
# Ask the user for their name and the year they were born.
name_prompt = "Please enter your name: "
birth_year_prompt = "Please enter your year of birth: "
current_year = 2018
# Step 2
# Calculate and print the year they'll turn 25, 50, 75, and 100.
# Step 3
# If they're already past any of these ages, skip them.
active = True
while active:
name = input(name_prompt)
birth_year = input(birth_year_prompt)
birth_year = int(birth_year)
current_age = current_year - birth_year
twenty_five = birth_year + 25
fifty = birth_year + 50
seventy_five = birth_year + 75
one_hundred = birth_year + 100
if int(current_age) < 25:
print("\n" + name.title() + ", you will turn 25 during the year " + str(twenty_five) + ".")
elif int(current_age) > 25:
active = False
if int(current_age) < 50:
print("\n" + name.title() + ", you will turn 50 during the year " + str(fifty) + ".")
elif int(current_age) > 50:
active = False
if int(current_age) < 75:
print("\n" + name.title() + ", you will turn 75 during the year " + str(seventy_five) + ".")
elif int(current_age) > 75:
active = False
if int(current_age) < 100:
print("\n" + name.title() + ", you will turn 100 during the year " + str(one_hundred) + ".")
elif int(current_age) > 100:
active = False
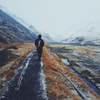
Akhil Purohit
Courses Plus Student 581 PointsNeed to keep practicing the for loop! wrote it in 16 lines until I saw Damien Watson code in 8 !
def age_app(): name = input("What is your name?: ") yob = int(input("What is your year of birth?: ")) twentyfive = yob + 25 fifty = yob + 50 seventyfive = yob + 75 hundred = yob + 100 if yob <= 1991.5: print("{} you are over 25!".format(name)) print("{} you will turn 50 in the year {}!".format(name, fifty)) print("{} you will turn 75 in the year {}!".format(name, seventyfive)) print("{} you will turn 100 in the year {}!".format(name, hundred)) elif yob >= 1992: print("{} you will turn 25 in the year {}!".format(name, twentyfive)) print("{} you will turn 50 in the year {}!".format(name, fifty)) print("{} you will turn 75 in the year {}!".format(name, seventyfive)) print("{} you will turn 100 in the year {}!".format(name, hundred))
age_app()
Damien Watson
27,419 PointsDamien Watson
27,419 PointsI also figured out you can get the current year with the following: