Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial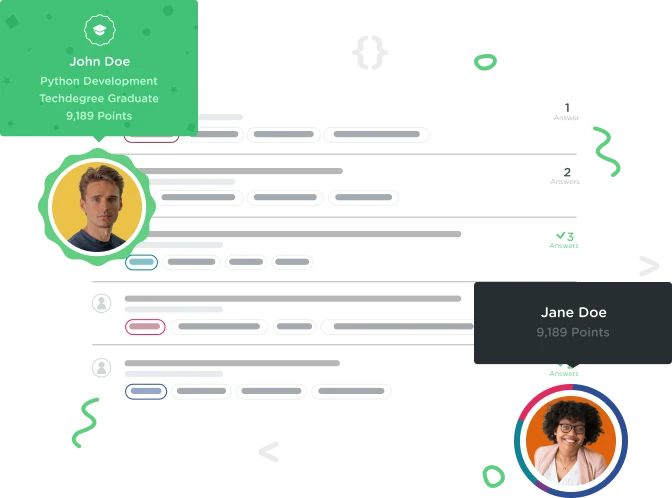
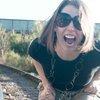
Beth Singley
3,491 Pointshow to iterate through two lists at the same time
Hi - I completed this challenge "combo" on tuples, but I don't understand what I did. I got stuck at trying to iterate through two lists at the same time. Below is the solution I found on the internet, but the "zip" function was definitely not in the lesson. When I didn't use "zip", I got an error about expecting two variables. Is there anyone out there with a different solution/explanation for how to step through two lists at the same time? I'd love to understand this more.
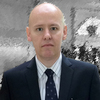
Nathan Tallack
22,159 PointsBeth, your code looks like it should work. Take care to verify that the indentation is as the mod has formatted it here. It may be a simple indentation error. ;)
Keep up the great work!
1 Answer
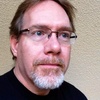
Chris Freeman
Treehouse Moderator 68,423 PointsTo fully use the power of zip
you can take advantage that it returns an interable that generates tuples. Wrapping that tuple generator in a list()
function get you back a list:
def combo (iter1, iter2):
return list(zip(iter1, iter2))
But that is way beyond where students are at this point. To solve it without using zip
you can walk down one or both of the iterables using an index:
# using general index
def combo(iter1, iter2):
new_list = []
for idx in range(len(iter1)):
new_list.append((iter1[idx], iter2[idx]))
return new_list
# using index on one iterable
def combo(iter1, iter2):
new_list = []
for idx, item in enumerate(iter1): # <-- returns index and one item from iter1
new_list.append((item, iter2[idx]))
return new_list
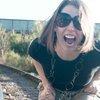
Beth Singley
3,491 PointsChris -
Thanks for your answer. I was looking for something that obviously used the lessons taught in the class. I see how the enumerate would provide an index and one item from iter1. Can you help me understand why you need the idx in the for loop? Does it have to do with the enumerate piece?
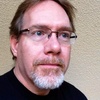
Chris Freeman
Treehouse Moderator 68,423 Pointsenumerate
is a useful function to loop through an iterable. For each item in the iterable, enumerate
returns a tuple of a the current loop count (starting with 0) and the next item from the iterable.
Since enumerate
returns a tuple of two items one needs to provide two variables to receive the count and the item from the iterable. I chose to use idx
to receive the index or count value.
Without using enumerate
you would need an explicit count to index into the second iterable.
# using a counter and looping through one iterable
def combo(iter1, iter2):
count = 0
new_list = []
for item in iter1:
new_list.append((item, iter2[count]))
count += 1
return new_list
More on enumerate
in the docs
Beth Singley
3,491 PointsBeth Singley
3,491 Pointsdon't know why my code didn't come through... here it is...
[MOD: Added ```python markdown formatting -cf]