Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial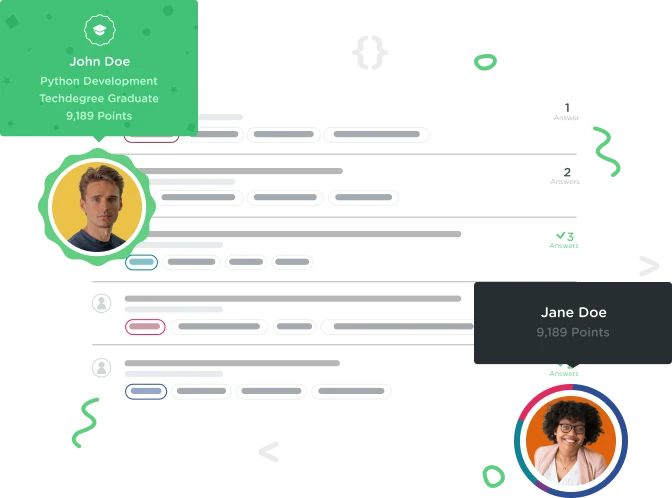

George Martinez
6,232 PointsHow to keep a variable as an integer in a constructor function?
Here is the code:
function Calculator (x,y) { this.x = parseFloat(x); this.y = parseFloat(y); this.adding = function() {console.log(x+y)}; this.subtracting = function() {console.log(x-y)}; this.multiplying = function() {console.log(x*y)}; this.dividing = function() {console.log(x/y)}; }
It's a constructor function for a rudimentary calculator object. I parseFloated the x and y parameters in order to convert them to floats. Everything works fine when you create a new Calculator, like: var calc = new Calculator(x,y);
BUT.......if I change the code INSIDE each of the console.logs to this:
function Calculator (x,y) { this.x = x; this.y = y; this.adding = function() {console.log(" Add " + x+y)}; this.subtracting = function() {console.log(" Subtract " + x-y)}; this.multiplying = function() {console.log(" Multiply " + x*y)}; this.dividing = function() {console.log(" Divide " + x/y)}; }
THEN the parsefloating function seems to be irrelevant because the JavaScript interpreter will assume that X and Y are STRINGS. The adding function will add 2 strings and the subtracting function will deliver a NaN result.
This is the question: How can I build a constructor function that maintains the Floats inside console.logs?
2 Answers
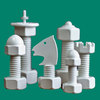
Steven Parker
231,248 PointsWhen you use the "+" symbol with a string, it's a concatenation operator. So if the next argument is a number, it is converted to a string and then tacked on. That's why adding returns all the values. But for subtracting, the "y" value is subtracted from the string made by the first concatenation, producing "NaN".
The solution is just to group the math operations to make sure they are performed first before any string conversions:
console.log(" Add " + (x + y));
// ...
console.log(" Subtract " + (x - y));
// etc...
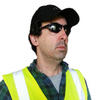
Phillip Kerman
Courses Plus Student 285 PointsI think all that's happening is that the + will create a string when EITHER (or both) its operands are strings. So, if do:
console.log("someString " + myNumber);
You get a string.
Just put your number variables in parenthesis to make them evaluate (as numbers) first... or you can always put them them in the parentheses of Number()

George Martinez
6,232 PointsThank you very much. The problem is solved. :-) I appreciate the time you took to answer my question.
George Martinez
6,232 PointsGeorge Martinez
6,232 PointsThank you very much. The problem is solved. :-) Thank you for your time. :-) Jorge