Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial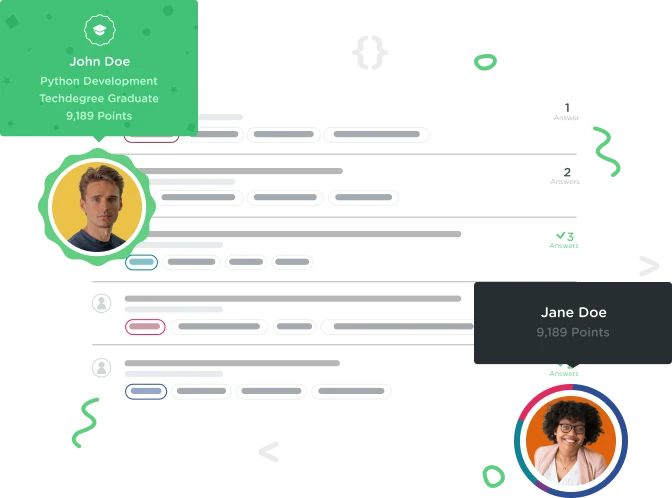

Julian Hurley
3,133 PointsHow to kill a javascript setTimeout loop.
I have this loop that works okay:
function countdown(counter) {
x = counter;
if (x > 0) {
x--;
alert(x + "/5");
if (x > 0) {
setTimeout(function () {
countdown(x);
}, 500);
}
}
};
countdown(6);
But I'd like to be able to kill it, and start it again by pressing a button. I'd like to restart the loop, regardless of what number it's on when I press the button.
I've got nowhere over the last few hours.
Here's a jsfiddle: http://jsfiddle.net/4vtPH/4/
Thanks a lot
1 Answer
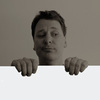
Sean T. Unwin
28,690 PointsTo end the setTimeout you're looking for clearTimeout(id)
. You will noticed the id
, this is where you have to assign a variable to the setTimeout for a reference.
Here's a fork of your fiddle with the changes I've made: http://jsfiddle.net/6ny8d/
Below is the code from the link above that I have added to work, I hope, in the way you would like and as well removed one of your if
statements. I have commented the code although feel free to ask any questions that you may have.
HTML:
<input type="button" id="button" value="reset count">
JAVASCRIPT:
/*
* @theTimer: we'll assign the setTimeOut function to this
* @total: counter number that's easily editable
* @btn: our button element
*/
var theTimer,
total = 5,
btn = document.getElementById("button");
/* Set the button's click event here to keep
* unneeded markup out of the HTML */
btn.addEventListener("click", function () {
countdown(total)
}, false);
/* Dynamically change button text based on @total */
btn.value = "Reset count to " + total;
function countdown(counter) {
/* Exit if nothing to do */
if (counter === 0) {
return;
}
/* Clear @theTimer */
clearTimeout(theTimer);
var x = counter;
if (x > 0) {
alert(x + "/" + total);
theTimer = setTimeout(function () {
countdown(x);
}, 2000);
x--;
}
}