Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial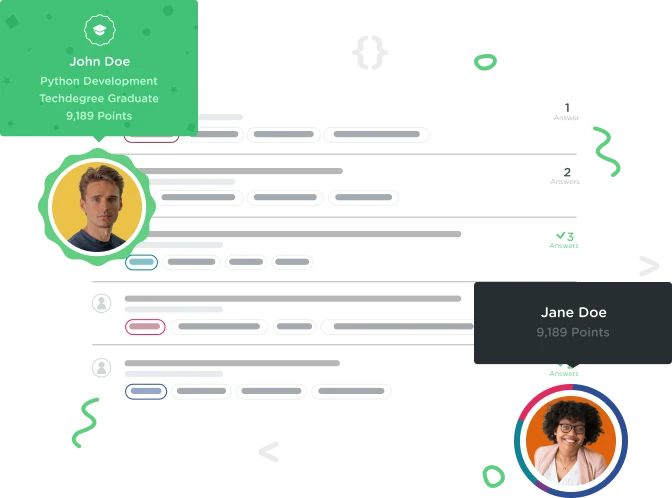

Anouk Lejeune
3,505 PointsHow to log out the even numbers from 2 to 24
I don't know how to target the even numbers:
for(var i=2;i<=24;i+=2){ if (i=== true) { i+=2; } console.log(); }
for(var i=2;i<=24;i+=2){
if (i=== true) {
i+=2;
}
console.log();
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
4 Answers
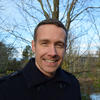
Stephen O'Connor
22,291 PointsYou shouldn't need the conditional as 'i' starts at 2 and iterates by 2 until it reaches 24.
for(var i = 2; i <= 24; i += 2){
console.log(i);
}
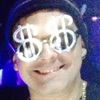
Mustafa Başaran
28,046 PointsHi Anouk,
You may want to try this, as well:
for (var i=2;i<=24;i+=1){ if (i % 2 === 0) { console.log(i); } }
% is the modulus operator. 'if' statement in the for loop writes the number, that is i, on the console only if i is divisible by 2. Of course, this can be extended to any case... for instance, in order to write down all numbers between 2 and 24 that are divisible by 6..
for (var i=2;i<=24;i+=1){ if (i % 6 === 0) { console.log(i); } }
I hope that this helps.

Anouk Lejeune
3,505 PointsHey Stephen and Mustafa,
Thank you both for the answers. In the meanwhile I got it resolved by searching on the internet. I found out about the way of Mustafa, with the modulus operator. I'm happy you wrote it down, because I didn't understand the complete meaning of (i % 2===0) (their was no explanation or name modulus operator where I found it) but with your answer I do.
Stephen, Your answer helped me in finding out that what I was doing at first was right, i just forgot to call for the i in console.log. The way i wrote it down above, was another solution, because at that time, I didn't see that I forgot the i, when at first, I just used the for loop, like you suggested. . So now I know it indeed was that simple.
So thank you to the both of you.
Greets,
Anouk
P.S: I wanted to give a 2 for best answer to the both of you, since both answers where spot on in what I needed. But apparently, you can't do that. Too bad, because it really should have been 2-2 instead of 1-1.
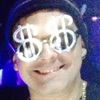
Mustafa Başaran
28,046 PointsThanks a lot for your kind reply, Anouk.
Jelena Feliciano
Full Stack JavaScript Techdegree Student 12,729 PointsJelena Feliciano
Full Stack JavaScript Techdegree Student 12,729 PointsThanks Stephen. I was stuck on this one too... I'm still not sure if I understand why this worked but I'm hoping with more practice I will understand.