Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial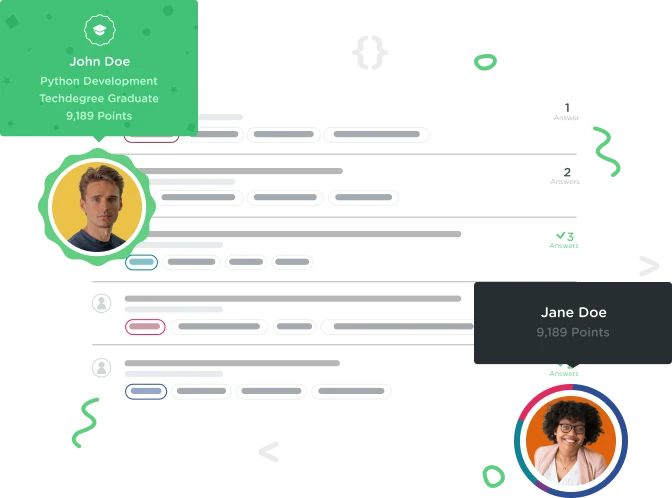
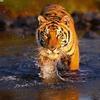
Konrad Pilch
2,435 PointsHow to make a flying umm, flying option.
HI,
I want to make flying here.
So if i press up arrow, it will just fly as long as its pressed, and then if i stop it will go down, if i want to press it agian in the air, it will work. Heres what i got:
(function() {
var requestAnimationFrame = window.requestAnimationFrame || window.mozRequestAnimationFrame || window.webkitRequestAnimationFrame || window.msRequestAnimationFrame;
window.requestAnimationFrame = requestAnimationFrame;
})();
var
canvas = document.getElementById("myCanvas"),
ctx = canvas.getContext("2d"),
width = 1900,
height = 1200,
player = {
x : width/2,
y : height - 5,
flyY : 3,
width : 70,
height : 70,
speed: 3,
velX: 6,
velY: 10,
fly: 5,
jumping: false,
flying: false
},
keys = [],
friction = 0.8,
gravity = 0.4;
canvas.width = width;
canvas.height = height;
function update(){
// check keys
if (keys[32]) {
//or space
if(!player.jumping){
player.jumping = true;
player.velY = -player.speed*4.4;
}
}
//up arrow
if (keys[38]) {
if(!player.flying) {
player.velY = player.speed-2;
}
}
//down arrow
if (keys[40]) {
player.velY = +player.speed*2;
}
//moving left
if (keys[37] || keys[30]) {
player.velX--;
}
//moving right
if (keys[39] || keys[33]) {
player.velX++;
}
player.velX *= friction;
player.velY += gravity;
player.x += player.velX;
player.y += player.velY;
if (player.x >= width-player.width) {
player.x = width-player.width;
} else if (player.x <= 0) {
player.x = 0;
}
if(player.y >= height-player.height){
player.y = height - player.height;
player.jumping = false;
}
ctx.clearRect(0,0,width,height);
ctx.fillStyle = "red";
ctx.fillRect(player.x, player.y, player.width, player.height);
requestAnimationFrame(update);
}
document.body.addEventListener("keydown", function(e) {
keys[e.keyCode] = true;
});
document.body.addEventListener("keyup", function(e) {
keys[e.keyCode] = false;
});
window.addEventListener("load",function(){
update();
});
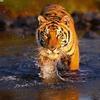
Konrad Pilch
2,435 Points<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>My Little game</title>
<script src="myscripts.js"></script>
<style type="text/css">
body{
background: #888;
}
canvas{
background: #222;
}
</style>
</head>
<body>
<canvas id="myCanvas"></canvas>
<script src="myscripts.js"></script>
</body>
</html>
Im drawing with canvas.
1 Answer
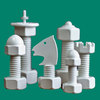
Steven Parker
231,198 PointsI finally got around to looking at this again (I've been answering several of your other questions meanwhile!).
It looks like you're missing a negative sign on line 45. Here it is corrected:
player.velY = -player.speed - 2;
Steven Parker
231,198 PointsSteven Parker
231,198 PointsIs there some HTML to go with this?