Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial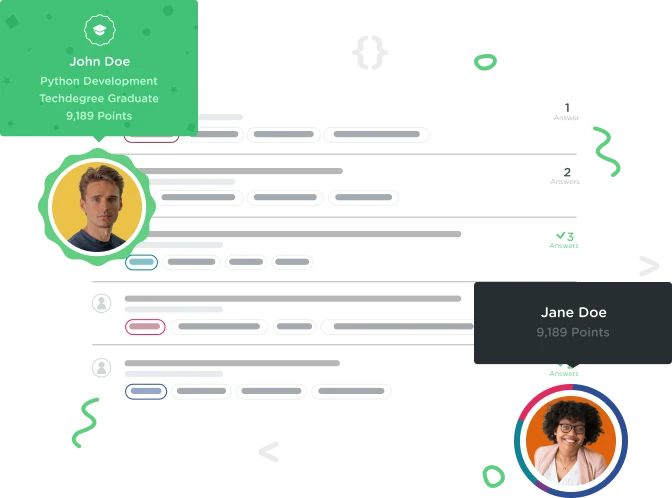

Julian Betancourt
11,466 PointsHow to make a light box go away on click ? JS
Hi guys
So I have a lightbox:
<!--This is generated with JS -->
<div class="overlay">
<div class="add-task-container">
<input type="text">
<button class="add-overlay">Add</button>
</div>
</div>
I want to make it disappear when everything but the input element is clicked. right now If the user clicks the input, the entire overlay will go away:
var addButtonCard = document.getElementById('add');
addButtonCard.onclick = lightBox;
function lightBox() {
var overlay = document.createElement('div');
overlay.className = 'overlay';
var section = document.getElementById('main');
section.appendChild(overlay);
var addTaskContainer = document.createElement('div');
addTaskContainer.className = 'add-task-container';
overlay.appendChild(addTaskContainer);
var addTextInput = document.createElement('input');
addTextInput.setAttribute('type', 'text');
addTaskContainer.appendChild(addTextInput);
var addButtonOverlay = document.createElement('button');
addButtonOverlay.className = 'add-overlay';
addButtonOverlay.innerHTML = 'Add';
addTaskContainer.appendChild(addButtonOverlay);
overlay.onclick = function () {
this.style.display = 'none';
}
addTextInput.onclick = function () {
overlay.style.display = 'flex';
}
//console.log(section);
}
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Julian,
It sounds like you have an event bubbling problem. The click event will normally bubble up through all the ancestors of the element that was clicked on. If any of those ancestors have a click handler then it will be triggered. So in your case, the handler for the text input is run and then the handler for the overlay is run.
You can stop the event bubbling with stopPropagation()
which can be called on an event object. This event object can be passed into your handler.
Try updating your code with the following and see if that fixes your problem.
addTextInput.onclick = function (event) {
overlay.style.display = 'flex';
event.stopPropagation(); // this prevents the click event from bubbling further up the chain
}
This should make it so that a click on the input only runs the handler for the input. A click anywhere on the div but outside the text input should only run the handler for the div.