Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial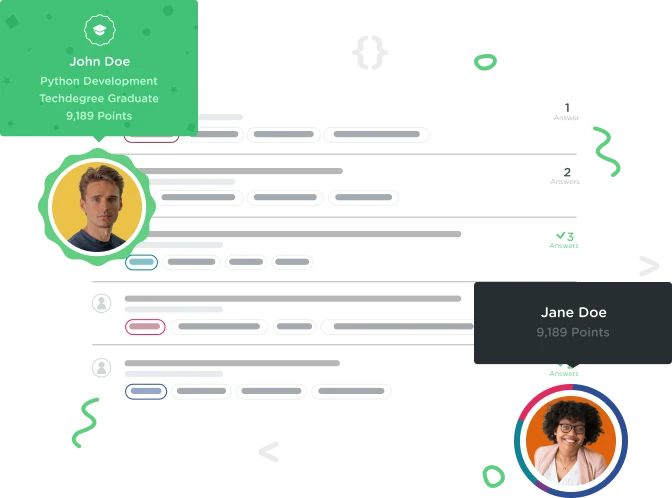

Xavier Downing
Courses Plus Student 1,339 PointsHow to make a method call a method that contains more than one parameter?
Hi. I think I found a bug in the iOS Syntax Overview video. In this video, its explained how you can chain multiple parameters to a method. Thus, an instance method with two parameters in the .h and .m files called,
-(void)setCenter:(NSArray *)center radius:(float)radius;
in order to replace two instance methods each containing one parameter, which are,
-(void)setCenter:(NSArray *)center;
, and,
-(void)setRadius:(float)radius;
.
However, in the main file, the method call was left unchanged to the one-parameter set-radius method, which is,
[ball setRadius:25]; // method call to -(void)setRadius:(float)radius
.
Now this method call works in the video and the code compiles successfully because he did not remove or comment out the associated method for the call. Thus the method call in the main file will still work because of its associated one-parameter method, and not on the new method with two parameters. The associated one-parameter method is one of the two that I stated above, which again is,
-(void)setRadius:(float)radius; // use [ball setRadius:25] to call to this method
.
Being that I decided to comment out the one parameter methods in order to use the method with multiple parameters, the setRadius call inside main no longer works being that the new method is not called setRadius, but is actually called,
-(void)setCenter:(NSArray *)center radius:(float)radius; // How do you make a call to this method?
The video gave an example of how to chain parameters to a method, but I’m not sure if he successfully provided an example of how to make a call to a method with multiple parameters. Is it possible to make a call to one parameter of a method with multiple parameters, or if I am to make the call, I have to call to ALL parameters of that method? Also, can I chain parameters to "getter" methods, and if so, how is that created and and how do I make calls to "getter" methods with multiple parameters?
Now that I removed the two one-parameter methods, I get an error in the main file stating “No visible @interface for ‘Sphere’ declares the selector ‘setRadius:’
I tried to guess the proper call to the method using,
[ball setCenter: radius:25]; // this call does not work on -(void)setCenter:(NSArray *)center radius:(float)radius, neither does [ball setRadius:25]
, but that failed with an error of, ‘Use of undeclared identifier ‘radius’. I guess that’s because the identifier is not “radius”, but “setCenter radius”.
All in all, I'm providing the entire code I have just in case I have something wrong in it that I may have missed, which really could be causing my problem.
First the .h file,
#import <Foundation/Foundation.h>
@interface Sphere : NSObject
{
NSArray *_center;
float _radius;
}
/*
-(void)setCenter:(NSArray *)center; */ // setter for center -- replaced by method with more than one parameter
-(NSArray *)center; //getter for center
/*
-(void)setRadius:(float)radius; */ // setter for radius -- replaced by method with more than one parameter
-(float)radius; //getter for radius
-(void)setCenter:(NSArray *)center radius:(float)radius;
@end
.
Now here's the .m file,
#import "Sphere.h"
@implementation Sphere
/*
-(void)setCenter:(NSArray *)center
{
_center = center;
} */ // setter for center -- replaced by method with more than one parameter
-(NSArray *)center
{
return _center;
} //getter for center
/*
-(void)setRadius:(float)radius
{
_radius = radius;
} */ // setter for radius -- replaced by method with more than one parameter
-(float)radius
{
return _radius;
} //getter for radius
-(void)setCenter:(NSArray *)center radius:(float)radius;
{
_center = center;
_radius = radius;
}
@end
.
And finally, the main file with the call to the method of one parameter instead of having a call to the method of multiple parameters,
#import <Cocoa/Cocoa.h>
#import "Sphere.h"
int main()
{
Sphere *ball = [ [ Sphere alloc ] init ];
[ball setRadius:25];
NSLog( @"The radius of ball is %f", [ball radius] );
return 0;
}
.
Please let me know if the bug I found is true and how to fix. Thanks a bunch!
1 Answer
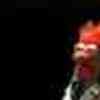
James Andrews
7,245 PointsIn your code center:(NSArray*) center
means that it is expecting an NSArray for the argument center. You are passing an int, so it should be center:(int):center
.

Xavier Downing
Courses Plus Student 1,339 PointsHi James,
From your answer, I guess you want me to conform the ‘methods’ in my .h and .m files, to the ‘method call’ in my main file, which is:
[ball setCenter: 34 radius: 25];
.
Now, if I do that, I will have to remove all of the 'NSArray' types, and replace them with 'int' types (or 'float' types since 'int type variables' can be 'float' types as well...but I'll do 'int' to make the 'parameter types' mixed), I’m going to do that now. First the .h file:
#import <Foundation/Foundation.h>
@interface Sphere : NSObject
{
float _radius;
int _center;
}
/*
-(void)setCenter:(NSArray *)center; */ // setter for center -- replaced by method with more than one parameter
-(int)center; //getter for center
/*
-(void)setRadius:(float)radius; */ // setter for radius -- replaced by method with more than one parameter
-(float)radius; //getter for radius
-(void)setCenter:(int)center radius:(float)radius;
@end
.
Now here’s the .m file:
#import "Sphere.h"
@implementation Sphere
/*
-(void)setCenter:(NSArray *)center
{
_center = center;
} */ // setter for center -- replaced by method with more than one parameter
-(int)center
{
return _center;
} //getter for center
/*
-(void)setRadius:(float)radius
{
_radius = radius;
} */ // setter for radius -- replaced by method with more than one parameter
-(float)radius
{
return _radius;
} //getter for radius
-(void)setCenter:(int)center radius:(float)radius;
{
_center = center;
_radius = radius;
}
@end
.
And lastly, the main file with NSLog printing out the center 'int':
#import <Cocoa/Cocoa.h>
#import "Sphere.h"
int main()
{
Sphere *ball = [ [ Sphere alloc ] init ];
[ball setCenter: 34 radius: 25];
NSLog( @"The radius of ball is %f", [ball radius] );
NSLog( @"The center of ball is %d", [ball center] );
return 0;
}
.
The code compiles successfully with no errors and generates an output of:
2014-06-28 14:00:28.529 MyFirstObjectiveCProgram[13219:303] The radius of ball is 25.000000
2014-06-28 14:00:28.544 MyFirstObjectiveCProgram[13219:303] The center of ball is 34
.
Great! Now I know how making a call to a 'method' with multiple parameter’s works, and I also know that I can make a 'method call' to a 'method' containing mixed parameter types. However, I did not want to conform the methods to the 'method call' (I want just the opposite, which is to conform my 'method call' to the 'method'). I want to know how to make a call to the following 'method' as it is shown here:
-(void)setCenter:(NSArray *)center radius:(float)radius;
.
The reason why I want to know how to make a call to this particular 'method' shown above is because the video used it as one of its examples. The video used it as in example of how to chain two parameters to one 'method' instead of making two individual 'methods' each with one parameter. From the video, I got the impression that the 'method' containing the 'NSArray' parameter type will work once the code compiles and runs. However, I realized that it does not work with the 'method call' in the main file, which is:
[ball setRadius:25];
.
The 'method' containing the 'NSArray' parameter type will not work with the above ‘method call’ because the ‘method call’ is not calling that 'method’s' name, which is, ‘setCenter radius’, but to the 'method' named, ‘setRadius.’ The reason why the code compiles and runs in the video is because the ‘setRadius’ 'method' was not removed from the code…it stayed within the code, and it is as follows:
-(void)setRadius:(float)radius; // setter for radius that works with [ball setRadius:25]; method call
.
Once I commented out the ‘setRadius’ 'method' (shown above), its ‘method call’ did not work anymore. I commented out the ‘setRadius’ 'method' to really see how the ‘setCenter radius’ 'method' (which contains the 'NSArray' parameter type) works, and in order to get it to work properly, I need to know how to properly call to it. Thus, I experimented with various ‘method calls’, such as the one below that you suggested that I should conform the methods to…:
[ball setCenter: 34 radius: 25];
.
The only reason why I used the 34 ‘int’ (which also can be 34 'float') in the call above was just to experiment and figure out how to make the right 'method call'. But like you implied, the 'method’s' first parameter is of type ‘NSArray’, not of type ‘int’. Thus in order for the code to work, I either have to change the first parameter of the 'method' from ‘NSArray’ to ‘int’ (as you suggested, or even to 'float'), or I have to figure out the right 'method call' to this 'method', which contains a 'NSArray' and a 'float'.
Xavier Downing
Courses Plus Student 1,339 PointsXavier Downing
Courses Plus Student 1,339 PointsI decided to continue to play around with the method call, and I have determined that I have to address all the parameters of the method that I am calling to. Thus, I tried to call to the method using,
[ball setCenter: 34 radius: 25];
Now instead of getting the no visible interface error, I now get an error stating, "Incompatible integer to pointer conversion sending 'int' to parameter of type 'NSArray'". I guess I now got right method call to the method with the multiple parameters by address all the parameters, but since the center parameter is of type NSArray, and I used an int in my call to it, thus the "Incompatible integer to pointer conversion...'" error is created.
I guess my next question is how to address (i.e. make a method call) to all parameters of a method that contains mixed types? The method with the two parameters has a type float and a type NSArray. I see how to address the float parameter, but how to address the NSArray parameter of that method in my method call, being that my current error message shows that I addressed it wrong? Below is my modified main file showing my call to both parameters: