Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial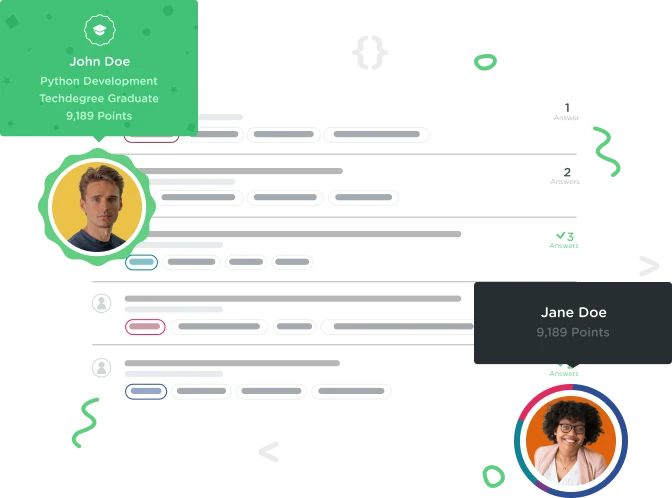
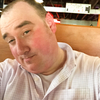
Victor Curtis Jr
5,268 PointsHow to make a print statement to test the game class?
So I was just following the video for making a matching card game in python and the instructor would like us to make a print statement to test the game class. She would like us to print out the rows and columns of the matching game. I just am unsure of exactly how to do this. I kinda put what I thought it would be, but it does not seem to print out anything. I will put what I have for the classes below.
class Card:
def __init__(self, word, location):
self.card = word
self.location = location
self.matched = False
def __eq__(self, other):
return self.card == other.card
def __str__(self):
return self.card
from cards import Card
import random
class Game:
def __init__(self):
self.size = 4
self.card_options = ['Add', 'Boo', 'Cat', 'Dev',
'Egg', 'Far', 'Gum', 'Hut']
self.columns = ['A', 'B', 'C', 'D']
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
self.locations.append(f'{column}{num}')
def set_cards(self):
used_locations = []
for word in card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_locations)
card = Card(word, random_location)
self.cards.append(card)
for num in range(1, self.size + 1):
print(f'{column}{num}')
if __name__ == '__main__':
Game()
1 Answer

jb30
44,806 PointsWhile you have defined a set_cards
method, you have not actually called it. Unlike __init__
, the set_cards
method is not run automatically when you create a new Game
instance. You could add the line self.set_cards()
to the end of the __init__
method to call the set_cards
method.
In the set_cards
method, used_locations.append(random_locations)
should be used_locations.append(random_location)
without the s
.
In the innermost for loop of the set_cards
method,
for num in range(1, self.size + 1):
print(f'{column}{num}')
the value for column
has not been declared. If the value of column
was A
, the set_cards
method would print
A1
A2
A3
A4
sixteen times. To instead print the value of the cards, you could try something like
for column in self.columns:
for num in range(1, self.size + 1):
for card in self.cards:
if (card.location == f'{column}{num}'):
print(card, end='')
print()
at the same indentation level as for word in card_options:
. This will loop through the list of cards
at each location and check if the card's location matches the current location. Using end=''
in the print
method will prevent the default line break. Using print()
after all the cards in a row are printed will give a line break.