Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial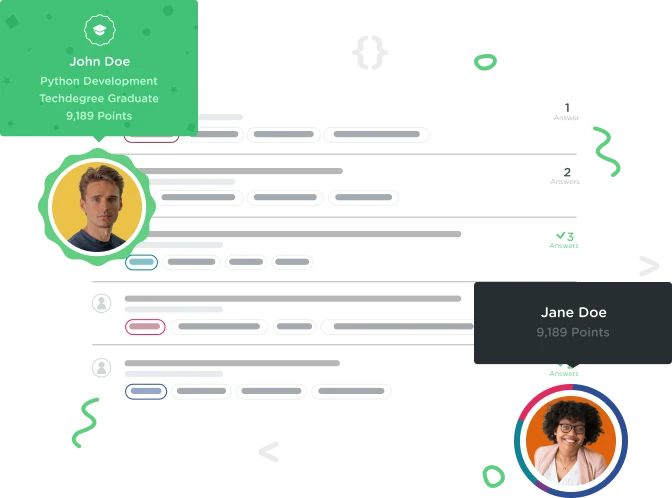
seif amr
324 Pointshow to make an image move up and down and keep moving up and down, on Xcode ?
i want to move an image up and down, but all i can do is to move it either up or down only. what can i do?
1 Answer

Nick Sint
4,208 PointsThere are 2 possibilities I see for this. Both use properties of UIView which can be found here: https://developer.apple.com/library/ios/documentation/uikit/reference/uiview_class/uiview/uiview.html
Option 1 - animateWithDuration:animations:completion:
Assuming you want to create some sort of oscillating effect (i.e. up and down forever), there will be two positions for the image, position A and position B. Here you would create a method that would essentially loop itself between A and B.
-(void)oscillation{
CGPoint PosA = CGPointMake(160, 50); //Assume Position A is at [160,50]
CGPoint PosB = CGPointMake(160, 300); //Assume Position B is at [160,300]
CGPoint newPos; //Define new position
if (CGPointEqualToPoint(imageView.center, PosA)){
newPos = PosB; //If the imageView is at Position A, set the new position at Position B
} else if (CGPointEqualToPoint(imageView.center, PosB)){
newPos = PosA; //If the imageView is at Position B, set the new position at Position A
}
//Animate the imageView to move to the new position and take 2 seconds to do it. Once done, call the oscillation method again
[UIView animateWithDuration:2.0 animations:^{
imageView.center = newPos;
} completion:^(BOOL finished) {
[self oscillation];
}];
}
Other variants of this method can be tried of course but it is usually handy if you want to animate different objects at different in different orders.
Option 2 - animateWithDuration:delay:options:animations:completion:
This second option simply uses 1 of UIView's class methods. You don't actually need to call/create a new method with this option.
...
CGPoint PosA = CGPointMake(160, 50); //Assume Position A is at [160,50]
CGPoint PosB = CGPointMake(160, 300); //Assume Position B is at [160,300]
CGPoint newPos; //Define new position
if (CGPointEqualToPoint(imageView.center, PosA)){
newPos = PosB; //If the imageView is at Position A, set the new position at Position B
} else if (CGPointEqualToPoint(imageView.center, PosB)){
newPos = PosA; //If the imageView is at Position B, set the new position at Position A
}
[UIView animateKeyframesWithDuration:2.0 delay:0.0 options:UIViewAnimationOptionAutoreverse | UIViewAnimationOptionRepeat animations:^{
[UIView setAnimationRepeatAutoreverses:YES]; //This ensures the animation not only animations forwards but back to its original position
[UIView setAnimationRepeatCount:100]; //Set the number of times you want the animation to repeat
imageView.center = newPos; //Define the new position for the imageView
} completion:^(BOOL finished) {
}];
...
Hope this helps!