Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial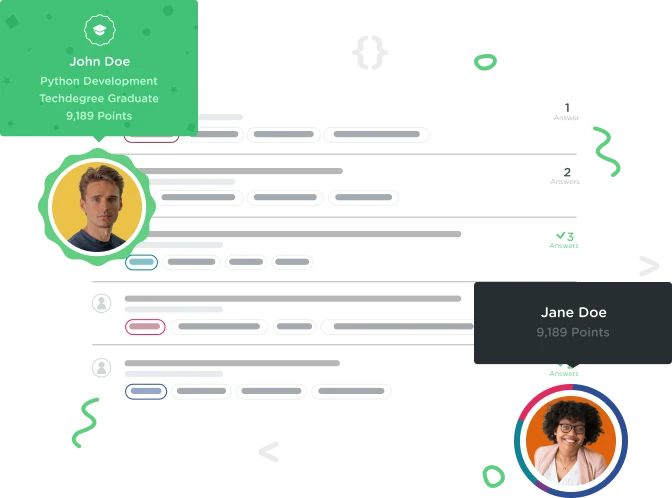

Camray Austin
Courses Plus Student 725 PointsHow to make code print("this item is not in your shopping list")? Having trouble with this really thought it was easy.
import os
shopping_list = []
def clear():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
clear()
# print out instructions on how to use the app
print("What items do you wish to add? ")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see current list.
Enter 'REMOVE' to delete a item from your list.
""")
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should i add {}?\n"
"Press ENTER to add to the end of the list\n"
"> ".format(item))
else:
position = 0
try:
postion = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(postion-2,item)
else:
shopping_list.append(new_item)
show_list()
def show_list():
clear()
print("Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print("-" * 10)
def remove_from_list(item):
show_list()
delete =input('What do you want to delete?\n> ')
try:
shopping_list.remove(delete)
except ValueError:
pass
show_list()
show_help()
while True:
new_item = input("> ")
# be able to quit the app
if new_item.upper()== 'DONE' or new_item.upper() == 'QUIT':
break
elif new_item.upper() == "HELP":
show_help()
continue
elif new_item.upper() == "SHOW":
show_list()
continue
elif new_item.upper() == 'REMOVE':
remove_from_list()
else:
add_to_list(new_item)
show_list()
3 Answers
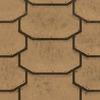
Wesley Trayer
13,812 PointsIn the "remove_from_list" function, after the
delete =input('What do you want to delete?\n> ')
add
if delete in shopping_list:
indent the try block and add an else to the if statement after the try block
else:
print("this item is not in your shopping list")

Mathew V L
2,910 PointsSorry for the old response, but this doesn't work for me. This is my code:
def remove_from_list():
show_list()
what_to_remove = input("What items do you want to remove you're list?")
if what_to_remove in shopping_list:
try:
shopping_list.remove(what_to_remove)
else:
print("Not Found")
I keep getting indentation error, any help would be great!
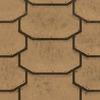
Wesley Trayer
13,812 PointsHey Mathew V L, an indentation error often occurs if the indentation is not consistent throughout the code. I would check the "spaces" option in the bottom right corner of workspaces, assuming you're using workspaces, it is usually set to "spaces: 4". If it is set to something else, say "spaces: 2" or "tabs 4", and it was set to "spaces: 4" while you were writing the rest of the code, it will throw an indentation error.
Also, I can't see the indentation of your code, because of the way it's formatted. If you want your code to look like it does in workspaces,
"Like this!"
check out the "Code" section of the "Markdown Cheatsheet".
Hope this helps!

Camray Austin
Courses Plus Student 725 PointsI removed that part.
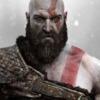
boi
14,242 PointsBro Camray (or sis).
Your code is perfectly fine, the only problem is that in the remove_from_list function you gave it an argument "item" which is invalid because you are asking for the argument from the user. The user will tell you what he whats to "remove" and that can be anything not necessarily an "item", that is why you used TRY block. Just write
def remove_from_list():
show_list()
delete =input('What do you want to delete?\n> ')
try:
shopping_list.remove(delete)
except ValueError:
pass
show_list()
and secondly, put your
delete = input('What do you want to delete?\n>')
in the TRY block like this, for better "beginner readability".
def remove_from_list():
show_list()
try:
delete =input('What do you want to delete?\n> ')
shopping_list.remove(delete)
except ValueError:
pass
show_list()
And that's done, your code is perfectly fine.
David Bath
25,940 PointsDavid Bath
25,940 PointsI don't understand your remove_from_list function. It uses a couple of variables that don't exist anywhere else in the code (word and secret_word).