Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial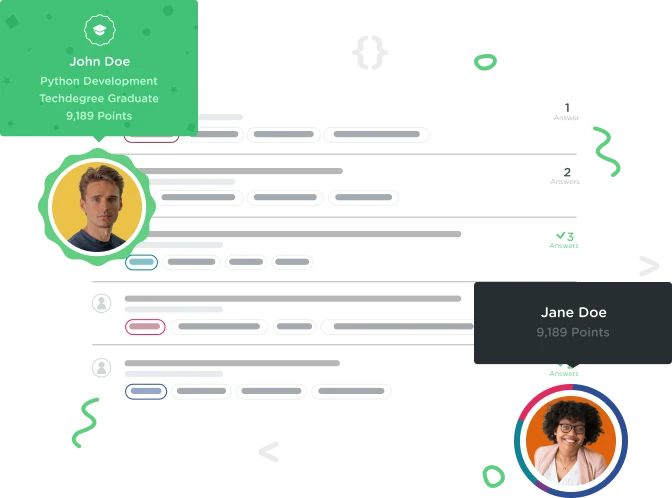
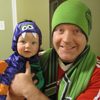
Brian Haucke
13,717 PointsHow to make network_unavailable_message into a dialog fragment
I'm stumped on this one. Can someone give me a nudge in the right direction? I'm thinking I'll be using alertUserAboutError(), but I don't know how to get the 'network_unavailable_message' to show up on the dialog. Thanks.
2 Answers

Mohammed Safiulla D
18,314 PointsYou can pass the message string when calling the alertUserAboutError method, then inside alertUserAboutError create a bundle with the string and pass this to the dialog (object of AlertDialogFragment) using setArgument(). So, depending on where you call the alertUserAboutError you get the network unavailable message string or the unsuccessful response string.
In the AlertDialogFragment receive this bundle using (getArguments()) and use the message from the arguments as the text you display in the dialog (builder.setMessage()).
So your code should look like,
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
else {
alertUserAboutError(getString(R.string.network_unavailable_message));
}
return isAvailable;
}
Modiy your alertUserAboutError() as the following:
private void alertUserAboutError(String message) {
AlertDialogFragment dialog = new AlertDialogFragment();
arg.putString("message_key",message);
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.setArguments(arg);
dialog.show(getFragmentManager(), "error_dialog");
}
Finally, in your AlertDialogFragment add get the bundle in the onCreate method:
public Dialog onCreateDialog(@Nullable Bundle savedInstanceState) {
Context context = getActivity();
Bundle bundle = getArguments();
String message = bundle.getString("message_key"));
AlertDialog.Builder builder = new AlertDialog.Builder(context);
builder.setTitle(R.string.error_dialog_title)
.setMessage(message);
builder.setPositiveButton(R.string.error_dialog_ok_button_text,null);
return builder.create();
}
Hope this helps.
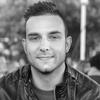
Pieter-Andries van der Berg
7,468 PointsThis is the correct method alertUserAboutError code:
private void alertUserAboutError(int message) {
AlertDialogFragment dialog = new AlertDialogFragment();
Bundle bundle = new Bundle();
bundle.putInt("message_key",message);
dialog.setArguments(bundle);
dialog.show(getSupportFragmentManager(),"error_dialog");
}
//and AlertDialogFragment:
public class AlertDialogFragment extends DialogFragment {
@NonNull
@Override
public Dialog onCreateDialog(@Nullable Bundle savedInstanceState) {
Context context = getActivity();
Bundle bundle = getArguments();
int message = bundle.getInt("message_key");
AlertDialog.Builder builder = new AlertDialog.Builder(context);
builder.setTitle(getString(R.string.error_title))
.setMessage(message);
builder.setPositiveButton(getString(R.string.error_button_ok_text),null);
return builder.create();
}
}
Brian Haucke
13,717 PointsBrian Haucke
13,717 Pointsprivate boolean isNetworkAvailable() {
}
private void alertUserAboutError() {
} }