Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial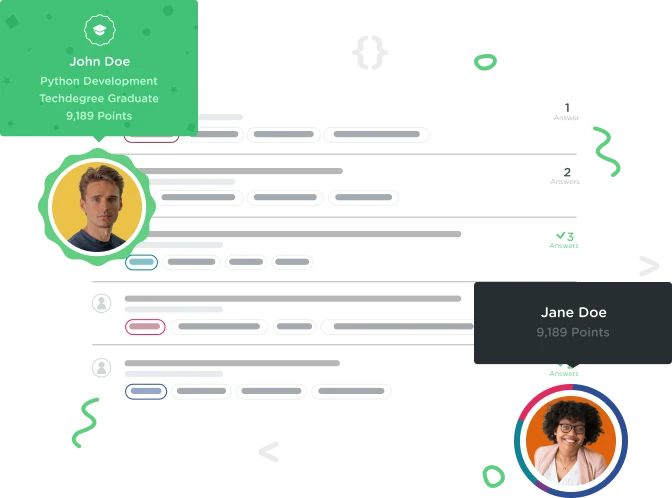
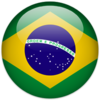
S Ahmad
228 PointsHow to make verify a friend request using Parse.com and How to verify the user using twilio
Hi,
I'd like help with two things if possible (both in relation to IOS apps). 1 - How can you (efficiently), create a 'friend request' element to the 'Ribbit' (snapchat) style app? Is there a clear and obvious way to verify friend requests that I'm missing? How would you do this using the parse.com framework?
2 - Using twilio, how can you verify the mobile number of someone in an IOS app? I would assume that when a user signs in, they give their mobile number. From here - I'm not too sure where to put the twilio code, I assume that the app will send a signal to run some code on a server, which will then send a text to your phone and update the database to have a matching number, and only when that number comes through is the app fully activated - is that the right way to think about it?
2 Answers

Stone Preston
42,016 Pointsfriend requests is a bit trickier than the current state of ribbit where you just add anybody you want. To implement something like friend requests (which requires modifying the current user thats accepting the request as well as the user who sent the request) you have to use Parse.com cloud code. Its ok. its just javascript but it can be kind of confusing. Ill walk you through the process of what its going to take to implement friend request.
You need to model a friend request in parse. So we need to create a friend request with a few attributes fromUser, toUser, and status. fromUser holds the user the friendRequest is from, toUser holds the user the request is to, and status holds the status of the request, accepted, pending, or denied.
currently you are just adding the user to the relation whenever you click on their name in the tableView that lists all the users. instead of that, we are going to create a new friend request object. so in cellForRowForIndexPath you should have something like
PFUser *selectedUser = [self.allUsers objectAtIndex:indexPath.row];
//request them
PFObject *friendRequest = [PFObject objectWithClassName:@"FriendRequest"];
friendRequest[@"from"] = self.currentUser;
//selected user is the user at the cell that was selected
friendRequest[@"to"] = selectedUser
// set the initial status to pending
friendRequest[@"status"] = @"pending";
[friendRequest saveInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {
if (succeeded) {
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Yay" message:@"Friend request sent" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil, nil];
[alert show];
} else {
// error occurred
}
}];
that creates the initial request. you could then add a tableView controller for friend requests that gets a users current pending requests that have been sent to him using a query:
//get the requests to the current user that are pending!
PFQuery *requestsToCurrentUser = [PFQuery queryWithClassName:@"FriendRequest"];
requestsToCurrentUser whereKey:@"to" equalTo:self.currentUser];
[requestsToCurrentUser whereKey:@"status" equalTo:@"pending"];
[requestsToCurrentUser findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if (!error) {
//this property stores the data for the tableView
self.friendRequests = objects;
//reload the table
[self.tableView reloadData];
} else {
//error occcurred
}
}];
the next part is the most difficult. When a user accepts a friend request, we need to add the user that sent the request to the currentUsers friends. but we also need to add the current user to the user who sent the requests friends as well. this is a problem since we cant modify users that arent logged in directly. You have to use cloud code.
to start with we are just going to call a cloud function that we havent written yet. when a user clicks on a cell, we want to accept the request. so in didSelectRowAtIndexPath you would need something like:
//get the selected request
PFObject *friendRequest = [self.friendRequests objectAtIndex:indexPath.row];
//get the user the request is from
PFUser *fromUser = [friendRequest objectForKey:@"from"];
//call the cloud function addFriendToFriendRelation which adds the current user to the from users friends:
//we pass in the object id of the friendRequest as a parameter (you cant pass in objects, so we pass in the id)
[PFCloud callFunctionInBackground:@"addFriendToFriendsRelation" withParameters:@{@"friendRequest" : friendRequest.objectId} block:^(id object, NSError *error) {
if (!error) {
//add the fromuser to the currentUsers friends
PFRelation *friendsRelation = [self.currentUser relationForKey:@"friends"];
[friendsRelation addObject:fromUser];
//save the current user
[self.currentUser saveInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {
if (succeeded) {
} else {
}
}
} else {
}
}];
so that calls the cloud code function named addFriendToFriendRelation. but we havent actually wrote that yet. follow the getting started guide to set up your cloud code on parse. after that, open the main cloud code file and add the function:
Parse.Cloud.define("addFriendToFriendsRelation", function(request, response) {
Parse.Cloud.useMasterKey();
var friendRequestId = request.params.friendRequest;
var query = new Parse.Query("FriendRequest");
//get the friend request object
query.get(friendRequestId, {
success: function(friendRequest) {
//get the user the request was from
var fromUser = friendRequest.get("from");
//get the user the request is to
var toUser = friendRequest.get("to");
var relation = fromUser.relation("friends");
//add the user the request was to (the accepting user) to the fromUsers friends
relation.add(toUser);
//save the fromUser
fromUser.save(null, {
success: function() {
//saved the user, now edit the request status and save it
friendRequest.set("status", "accepted");
friendRequest.save(null, {
success: function() {
response.success("saved relation and updated friendRequest");
},
error: function(error) {
response.error(error);
}
});
},
error: function(error) {
response.error(error);
}
});
},
error: function(error) {
response.error(error);
}
});
});
its not too complicated. the friend request id was passed in to the function as a parameter since you cant pass whole objects. we then query for that request. once we have it, we have access to the fromUser and toUser objects. we use those to add the acceptingUser to the fromUsers friends. then we save the fromUser and change the status of the friend request.
thats it. its kind of complicated I know. I actually just implemented this in an app im working on yesterday so im glad you asked
cant really help you with the second part of the question sorry.
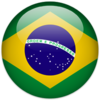
S Ahmad
228 PointsAh man thanks so much! I'll give it a go, probably will need a bit more help - thanks so much though!
Alex Reed
40 PointsAlex Reed
40 PointsCan you make an android versions of this please?
Vincent Jardel
6,436 PointsVincent Jardel
6,436 PointsThank you so much !!!