Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial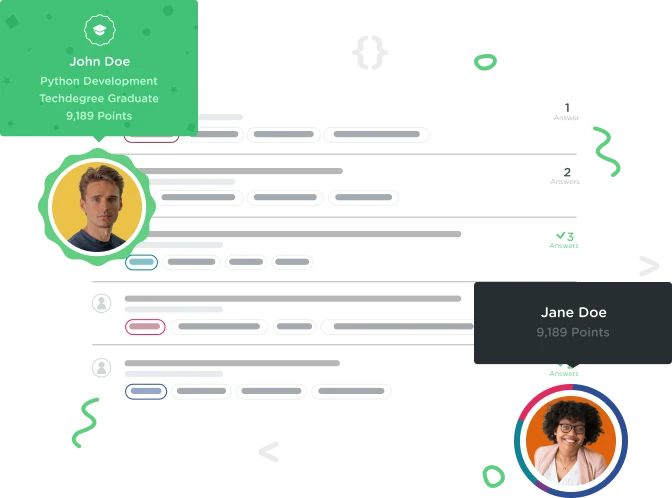
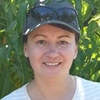
Olga Kireeva
9,609 PointsHow to minimize the use of global variables in JavaScript
Hello everybody! I’m learning coding in JavaScript and would like to apply best practices in my projects. I’ve learned that we should minimize the use of global variables in our programs and I understand why. My question is how we can do this. I know about the scope of variables and declaration of variables inside functions. However, sometimes I encounter the situation when I have to use the same variables in a few different functions.
1 Answer

Catherine Gracey
11,521 PointsI am working around this issue using three main approaches.
1 - A global object that contains most of my variables and functions. The benefit of this approach is that I can use consistent variable and function names across the different parts of my project. For example:
var modules = {
common: function(){
//Some code
},
mod1: {
var1: "string",
func1: function(){
//Some code
},
mod2: {
var1: "another string",
func1: function(){
//Some different code
}
};
2 - Passing values as arguments and returning values. Most of my functions could be rewritten to return a value rather than storing it globally. For example, instead of writing:
var randomNumber;
var range = 5;
function getRandomNumber(){//Get a random number between 0 and the value of range.
randomNumber = Math.floor(Math.random() * range);
}
I can write:
function getRandomNumber(range){
return = Math.floor(Math.random() * range);
}
and then store the result in a local variable within the function that calls it.
3 - Use container functions. Instead of having a sequence of variables and functions at the global level, wrap the whole process inside a function that can then store as many local variables as you like. For example:
function buildGameBoard(){
var someVar1, someVar2, asManyVariablesAsYouLike;
function func1(){
//Some code
}
function func2(){
//You get the idea
}
func1();
func2();
}
You can combine these approaches to make code that is very easy to navigate. In my own project I have a large global object named "modules" that has a section containing all of the code common between modules, and a section to contain each module. Consistent naming patterns mean I can find anything I'm looking for in seconds, and I tend to make fewer mistakes because now my different functions behave like predictable methods.
Olga Kireeva
9,609 PointsOlga Kireeva
9,609 PointsThank you, Catherine! Your explanation is so clear, helpful and well-thought through. Now I just need to learn to put these approaches in practice.