Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial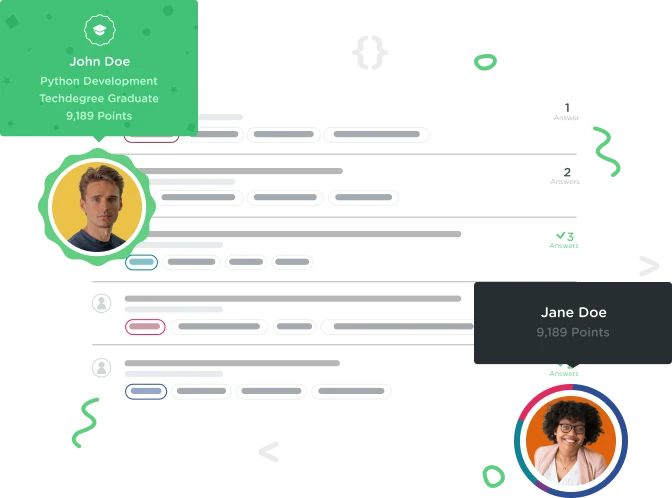
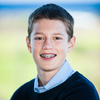
Edward Powley
515 PointsHow to modify the parameter
Here are the instructions... Modify the function named greeting to accept a parameter. Name the parameter person which is of type String.
func greeting(Hello) {
println("Hello")
}
2 Answers

Chuck Toussieng
1,214 PointsHey Edward.
The Apple docs are great for this kind of thing :)
func greeting(person: String) {
let myGreeting = "Hello, " + person + "!"
println(myGreeting)
}
If you want to make this a function that returns a string, you can do this:
func greeting(person: String) -> String {
let myGreeting = "Hello, " + person + "!"
return myGreeting
}
Then anywhere in your code you can call it like this:
println(greeting("Edward"))
And you will get "Hello, Edward!"
Try it in a Playground. Good Luck, and don't be afraid of the Docs, they are your friend.
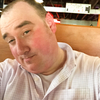
Victor Curtis Jr
5,268 PointsSo I had to go to Gbase "Google" for help with this question. What I found was some articles about swift that really helped give some insight for how to change the function and subsequent code so that it'd work. This is what I found:
func sayHello(personName: String) -> String { let greeting = "Hello, " + personName + "!" return greeting }
This is from an article about functions in swift. This is NOT the exact answer, but this should give you the necessary ideas for how to modify the function to get the green check!
Here is a link to the article: https://developer.apple.com/library/prerelease/ios/documentation/Swift/Conceptual/Swift_Programming_Language/Functions.html