Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial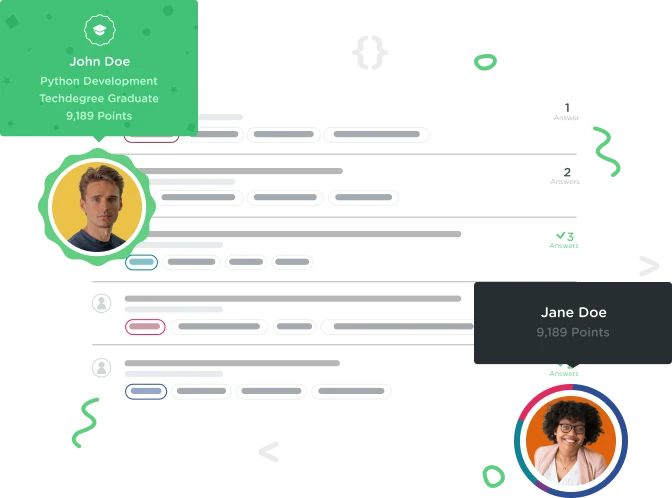
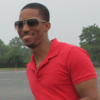
Michael Patterson
4,338 PointsHow to not add empty tasks
I just wanted to share with the community my solution for the above challenge.
---below code to be added in app.s---
//add task
//create task when button pressed
var addTask = function() {
console.log("Add Task");
//create task entered by user
var listitem = creatednewtask(inputTask.value);
//add to list only if value not null
if(inputTask.value) {
incomTaskHolder.appendChild(listitem);
bindEvents(listitem,compTask);
}
//inputTask is variable for new-task which we created in beginning
//since appendChild "adds" to list, we simply call it only if inputTask has a value
//inputTask will be a different variable in your own script but hopefully the point is clear
//assigning empty string after input
inputTask.value = '';
}
7 Answers
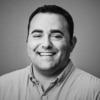
mikes02
Courses Plus Student 16,968 PointsIt would likely be helpful in a situation like that to also inform the user why they weren't able to add, you could use an else clause with an alert, for example:
if(taskInput.value) {
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
} else {
alert("You must enter a task.");
}
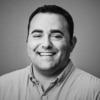
mikes02
Courses Plus Student 16,968 PointsJeremy,
Do you have your code to post? If you notice my code and Michael's code from above differ slightly I was just giving an example based on what I was using.
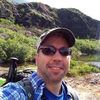
Jeremy Lindstrom
6,646 PointsHere's my complete app.js
//Problem: user interaction doesn't provide desired results
// Solution: Add interactivity so the user can manage daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton= document.getElementsByTagName("button")[0]; //first-button
var incompleteTasksHolder= document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder= document.getElementById("completed-tasks"); //completed-tasks
//New Task list item
var createNewTaskElement = function(taskString) {
//Create list item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox= document.createElement("input"); //checkbox
//label
var label = document.createElement("label"); //label
//input (text)
var editInput = document.createElement("input");
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//each element needs modifying
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
label.innerText = taskString;
// each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//Add a new task
var addTask = function() {
console.log("Add task...");
//create a list item with the text from #new-text
var listItem = createNewTaskElement(taskInput.value);
if(taskInput.value) {
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
} else {
alert("You must enter a task.");
}
//append listItem to incompleteTaskHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
taskInput.value = "";
}
//edit an existing task
var editTask= function(){
console.log("Edit task...");
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text]");
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode");
var editBtn = listItem.getElementsByTagName('button')[0];
//if the class of the parent is .editMode
if (containsClass) {
//switch from editMode
//label text become the input's value
label.innerText = editInput.value;
editBtn.innerText = "Edit";
} else {
//switch to .editMode
//input value becomes the label's text
editInput.value = label.innerText;
editBtn.innerText = "Save";
}
//Toggle .editMode on the list item
listItem.classList.toggle("editMode");
}
//Delete an existing task
var deleteTask = function() {
console.log("delete task...");
var listItem = this.parentNode;
var ul = listItem.parentNode;
ul.removeChild(listItem);
}
//mark a task complete
var taskCompleted = function() {
console.log("task complete...");
//append the task list item to the #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//mark a task incomplete
var taskIncomplete = function() {
console.log("task incomplete...");
//append the task list to the #uncompleted-tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select it's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to complete button
checkBox.onchange = checkBoxEventHandler;
}
//set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over incompleteTaskHolder ul list items
for (var i = 0; i < incompleteTasksHolder.children.length; i++) {
//bind events ot list items' children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completedTaskHolder ul list items
for (var i = 0; i < completedTasksHolder.children.length; i++) {
//bind events ot list items' children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
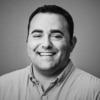
mikes02
Courses Plus Student 16,968 PointsI believe I see what's happening, you are adding regardless of the IF conditional.
if(taskInput.value) {
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
} else {
alert("You must enter a task.");
}
//append listItem to incompleteTaskHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
You have it within the IF conditional but also outside of it, so it's adding no matter what. You need to have it only within the IF conditional, like so:
if(taskInput.value) {
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
} else {
alert("You must enter a task.");
}
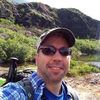
Jeremy Lindstrom
6,646 PointsAhh totally missed that! I've been looking at it for hours. :D kept glancing right over it. Thanks!
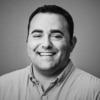
mikes02
Courses Plus Student 16,968 PointsI feel your pain, I've done that myself many times, it often helps to have a fresh set of eyes on it.
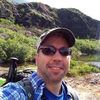
Jeremy Lindstrom
6,646 PointsWhen I use try the above code it still adds the empty item, and gives me the prompt if null.
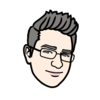
pauloliva
7,889 PointsThis was my solution... I don't create listItem unless it goes through my if statement.
// Add a new task
var addTask = function() {
console.log('Adding task...');
if(taskInput.value.length > 0) {
// Create a new list item with the text from #new-task:
var listItem = createNewTaskElement(taskInput.value);
// Append listItem to incompleteTaskHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
taskInput.value = '';
} else {
alert("You need to enter some text!");
}
}

Paul Kimberlee
5,634 PointsI just changed the addTask function to add an if statement that exits the function if the taskInput value == "" (equals empty string) . Not sure if this is the best way but it works fine.
like so.
var addTask = function(){ console.log("add task...");
if (taskInput.value == ""){return;}else{
var listItem = createNewTaskElement(taskInput.value);
//append to the incomplete tasks list
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem,taskCompleted);
taskInput.value ="";
}
}
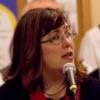
Tracy Trathen
10,468 PointsI followed along throughout the course, and so my code is pretty much like our instructor's code plus I've added the "Edit" vs "Save" as discussed at the end of the last video. Regarding the disabling of the "addTask" if the field is empty, I tried it both of the ways mentioned by others in this post (i.e. taskInput.value.length > 0 and the taskInput.value === "") but one issue I have found with this is that if you type a "space" in the input field, then click "add" it still creates the task. In other words, what you get is what looks like blank task with checkbox and the "edit" and "delete" buttons (just like before) when in reality it's a task with a "space" (or more spaces) in it.
I read a bunch on different ways to check for "spaces" (which is why I'm still up at 4am LOL) but I still don't know enough yet on how to actually implement a solution for that problem. I'd be interested to know how to check the input field for space(s) , and ONLY space(s) and if so, to not allow the "addTask" to run....
Ideas, help, comments, suggestions would be greatly appreciated. Thanks :)
eck
43,038 Pointseck
43,038 PointsI added some Markdown formatting to your post. If you want to share snippets of code in the forum the Markdown Cheatsheet is really helpful to get the best formatting.
You can find the cheatsheet it as a bold link just above the "Post comment" button.