Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial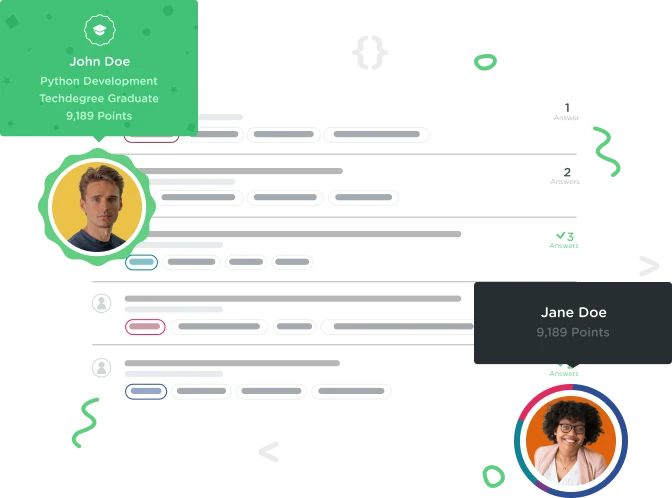
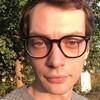
Tomasz Grodzki
8,130 PointsHow to open a file from disk using JavaScript?
Hi there!
I'm trying to open some file from my disk using a JS, and it doesn't work. I'm getting error because of CORS policy. This is my code. How can I bypass this problem?
function getRecipesFile () {
const recipeFile = new XMLHttpRequest();
recipeFile.open ( "GET", "przepisy.txt" );
recipeFile.onreadystatechange = function () {
if ( recipeFile.readyState === 4 ) {
if ( recipeFile.status === 200 || recipeFile.status === 0 ){
console.log ( recipeFile.responseText )
}
else {
console.log ( 'there was an error' );
}
}
}
recipeFile.send();
}
getRecipesFile();
3 Answers
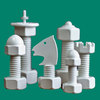
Steven Parker
231,271 PointsCORS prevents a page loaded from one server from accessing a page or other resource on a different server.
It is possible to overcome this restriction if both servers provide explicit permission by adding special headers to the HTML transmissions. For more details, see the MDN page on Cross-Origin Resource Sharing (CORS). But this should not impact resources coming from the same source.
It may be that the CORS mechanism is misinterpreting the URL as having a different origin. Try making the URL more specific.
If you still have trouble, you can make a snapshot of your workspace and post the link to it here, to allow the issue to be replicated and examined.

Kalen Honeyfield
Full Stack JavaScript Techdegree Graduate 17,718 PointsIf the file is being stored locally on disk, and you are using a normal unmodified browser (Chrome/FireFox/IE/etc) you won't be able to read that file using the method you've supplied. This is a security feature, and it prevents web pages from accessing your local hard drive.
Now, you have 2 options that I'm aware of.
- Option 1: Use a file handler and have user interaction provide the file https://web.dev/read-files/
- Option 2: Include the file in your header and assign the contents to a variable. <script src="przepisy.txt"></script>
If you're going to use option 2, just remember that its not a "live" connection to the file, and you'll have to refresh the page each time.
I'd recommend Option 1.
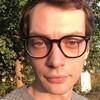
Tomasz Grodzki
8,130 PointsI'd like to make a page with recipes. It has to take json data from the file and convert this content to html using JS. I'd like to add an option to add some new recipes.
For my purpose the second option is better. But how can I access to the content of this json file?

Kalen Honeyfield
Full Stack JavaScript Techdegree Graduate 17,718 PointsYou wouldn't be able to add new recipes permanently, as the file is not "live". However you could add new data to that JSONObject.
The easiest method to get to the data is just declare is a variable like you would normally.
Let ingredients = {
"fruit": "Apple",
"size": "Large",
"color": "Red"
}
And now you can use ingredients just like you would normally. If you're looking for a more permanent solution I'd recommend a Node.js app with a database backend. But, this should at least get you started.
Steven Parker
231,271 PointsSteven Parker
231,271 PointsNow that I've seen the snapshot, I don't think you can load a JSON file in like that with a "script" tag. But you could get it with AJAX using "fetch", "XMLHttpRequest" or if you have jQuery, "getJSON".
But I didn't see any CORS errors running that snapshot.
Tomasz Grodzki
8,130 PointsTomasz Grodzki
8,130 PointsI updated the code. And every method (XMLHttpRequest, fetch and jQuery getJSON) gives me a CORS policy...
Steven Parker
231,271 PointsSteven Parker
231,271 PointsCan you post a fresh snapshot with the new code in it?
Tomasz Grodzki
8,130 PointsTomasz Grodzki
8,130 PointsAw, I was sure that I pasted it. My bad. There you go: https://w.trhou.se/uscz1we5jw
Steven Parker
231,271 PointsSteven Parker
231,271 PointsNo errors for me running the snapshot fork. My (chrome) console shows:
And jQuery is known for automatically overcoming CORS issues! Have you tried a different browser?
Tomasz Grodzki
8,130 PointsTomasz Grodzki
8,130 PointsOkay, it works if you are opening it through workspace. But it gives me a cors error when I'm working with files on my hard drive. What is the cause? Is there any chance to make it work?
Steven Parker
231,271 PointsSteven Parker
231,271 PointsWhere's the server that the page comes from?
Tomasz Grodzki
8,130 PointsTomasz Grodzki
8,130 PointsThere's no server, everything's on my hard drive.
Steven Parker
231,271 PointsSteven Parker
231,271 PointsThe browser is just reading your source files directly? Then what's performing the AJAX service request?
Tomasz Grodzki
8,130 PointsTomasz Grodzki
8,130 PointsYeah, probably reads the file directly and I wonder how to get to this file using JS. I've got 3 files in one folder (index.html, app.js, data.json). I'd like to get to the contents of data.json via JS.
Many people mostly recommended using $.getJSON or AJAX. Some time ago these methods were appropriate, but presently CORS doesn't allow doing that in this way. And I cannot find some good solution.
I think that, like Kalen said below, that I can use Node.js to do this. But... I wonder if I could do this using another tool?
Steven Parker
231,271 PointsSteven Parker
231,271 PointsAJAX (including jQuery's "getJSON") requires a server to handle the request. I'm not sure why you'd be getting a CORS reply unless the server has some rules that are being applied first. Try giving the file a more explicit path (like "file://C:/your-folder-path/data.json". If my guess is right, that may stop the CORS message but then you might get some error about no server.
Having your own service would seem the best solution. It could be done in node, python, or any of several other languages. The fact that the browser will read page files directly is not intended to completely replace normal operation with a server.
Development systems (like Visual Studio) crank up their own servers for testing like the workspaces do, that might be a way to go also.
Tomasz Grodzki
8,130 PointsTomasz Grodzki
8,130 PointsOkay, I see. Now everything became much clearer. Thank you for explanation!
Take care!