Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial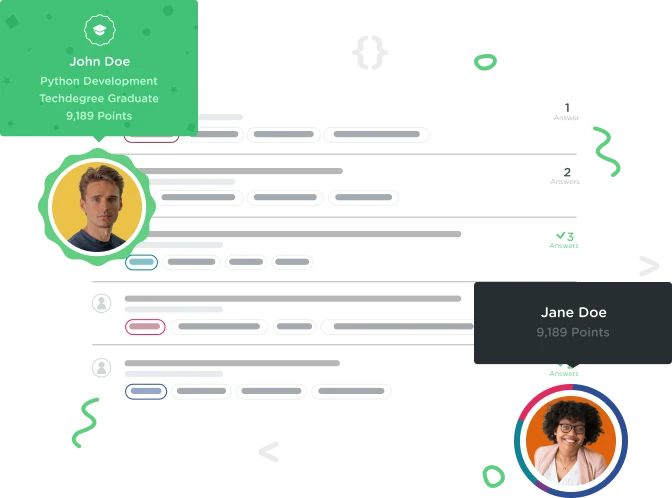

Sebastiaan van Vugt
Python Development Techdegree Graduate 13,554 PointsHow to override add-item properly
In the light of the exercise, I'm trying to override the add_item method with super. I have the feeling that I almost got it but apparently not entirely... My code is:
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def __init__(self, item):
self.slots = []
super().__init__()
super().add_item(item)
Any help is much appreciated.
P.S. Please note that the indentation of the last code line is off but that it is not in the actual code (this is a strange artefact)
2 Answers
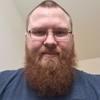
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsRemove the override of def init(self, item)
these might also make it fail (not sure though)
a = SortedInventory(Inventory)
a.add_item("Cookies")
a.add_item("Apple")
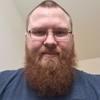
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsTo override add_item you would want to do something like this
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def __init__(self, item):
self.slots = []
super().__init__()
def add_item(self): #insert desired args
super().add_item(item)

Sebastiaan van Vugt
Python Development Techdegree Graduate 13,554 PointsFirst of all, thanks a lot. I think it works perfectly. However... the assignment is still not accepted and I do not understand why. My code is now:
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def __init__(self, item):
self.slots = []
super().__init__()
def add_item(self, item):
super().add_item(item)
a = SortedInventory(Inventory)
a.add_item("Cookies")
a.add_item("Apple")
The task was: "Now override the add_item method. Use super() in it to make sure the item still gets added to the list." Thank you for any additional ideas here.