Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial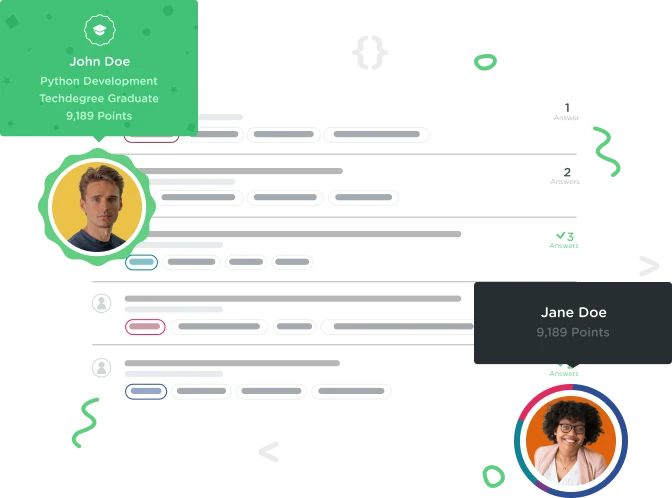

Benyam Ephrem
16,505 PointsHow To Parse JSON Using Jackson (Weird Scenario)
So I am creating an application that fetches data from the Alpha Vantage API and I am trying to parse this JSON response that can be seen here.
I have set up 3 Data Transfer Objects like such:
Stock.java
public class Stock extends Dto{
@JsonProperty("Time Series (Daily)")
private TimeSeries timeSeries;
public TimeSeries getTimeSeries() {
return timeSeries;
}
public void setTimeSeries(TimeSeries timeSeries) {
this.timeSeries = timeSeries;
}
}
TimeSeries.java
public class TimeSeries extends Dto{
private List<Date> dates;
public List<Date> getDates() {
return dates;
}
public void setDates(List<Date> dates) {
this.dates = dates;
}
}
Date.java
@JsonIgnoreProperties(ignoreUnknown = true)
public class Date extends Dto{
@JsonProperty("1. open")
private double open;
@JsonProperty("2. high")
private double high;
@JsonProperty("3. low")
private double low;
@JsonProperty("4. close")
private double close;
@JsonProperty("5. volume")
private double volume;
public double getOpen() {
return open;
}
public void setOpen(double open) {
this.open = open;
}
public double getHigh() {
return high;
}
public void setHigh(double high) {
this.high = high;
}
public double getLow() {
return low;
}
public void setLow(double low) {
this.low = low;
}
public double getClose() {
return close;
}
public void setClose(double close) {
this.close = close;
}
public double getVolume() {
return volume;
}
public void setVolume(double volume) {
this.volume = volume;
}
}
The field in Stock.java populates just fine since I explicitly say the name of the key and a value is accordingly parsed. But in the next step I want to store a List<Date> of objects that contain the information I'm going after and this does not work (returns null) because unless I explicitly give a key for an attribute Jackson just does nothing.
So I'm wondering how do I correctly parse this JSON in Java so that I can get an array of the dates and be able to access the properties of each date holding the information that I'm after?
1 Answer

Seth Kroger
56,413 PointsI think the main issue is that TimeSeries is a JSON object that uses the date as the key for data objects, not a JSON array. You would do best to place them in a Map than a List (or figure out if Jackson supports converting the key-value pairs into a list/array during parsing).
Benyam Ephrem
16,505 PointsBenyam Ephrem
16,505 PointsThanks! This helps alot!