Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial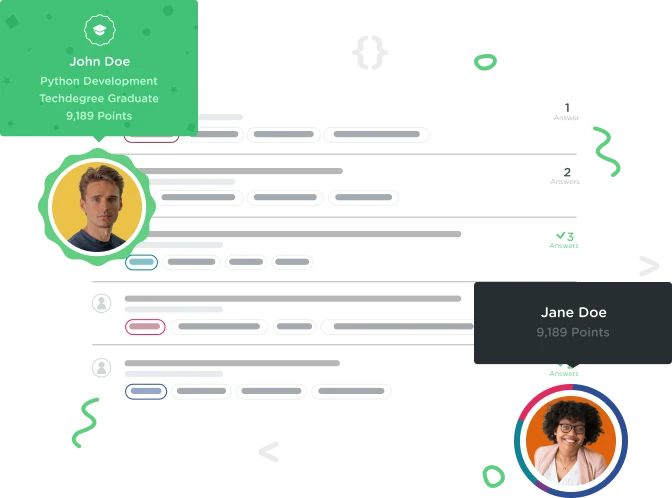
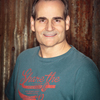
Bill Dowd
7,681 PointsHow to pass a variable to a modal from the button.
In the function below, I have included a button that triggers a modal. However, the button is part of a list that could have more than one result. I need to include the ID value of each database result in each button or modal trigger so that the value gets passed to a function call inside the modal body. How can I do this? Thanks, Bill
// For the Payee Dashboard
public function FormatPayeeDebtsTable($payee_id) {
$objVendor = new Vendor(0);
$form = "";
$form .= "<div class='row'>\n";
$form .= "<div class='col-md-12'>\n";
$form .= "<div class='tabletitle2'>Debts</div>\n";
$form .= "<table class='table-striped table-bordered' width='99%'>\n";
$form .= "<tr>\n";
$form .= "\t<th width='25%'>Vendor</th>\n";
$form .= "\t<th width='25%'>Opening<br />Balance</th>\n";
$form .= "\t<th width='25%'>Balance</th>\n";
$form .= "\t<th width='15%'>History</th>\n";
$form .= "\t<th width='5%'> </th>\n";
$form .= "\t<th width='5%'> </th>\n";
$form .= "</tr>\n";
if(empty($this->GetPayeeDebts($payee_id))) {
$form .= "<tr>\n";
$form .= "<td colspan='6'>There are no debts to display.</td>\n";
$form .= "</tr>\n";
} else {
$results = $this->GetPayeeDebts($payee_id);
foreach($results as $value) {
$this->id = $value['id'];
$this->opening_balance = $value['opening_balance'];
$form .= "<tr>\n";
$form .= "<td>" . $objVendor->GetVendorName($value['vendor_id']) . "</td>\n";
$form .= "<td>$" . number_format($value['opening_balance'], 2) . "</td>\n";
// $form .= "<td>$" . $this->GetDebtBalance(0) . "</td>\n";
$form .= "<td>$" . number_format(($this->GetDebtBalance($value['id']) == 0 ? $value['opening_balance'] : $value['opening_balance'] - $this->GetDebtBalance($value['id'])), 2) . "</td>\n";
$form .= "<td style='text-align: center;'><button type='button' class='btn btn-info btn-sm' data-toggle='modal' data-target='#myHistory' data-backdrop='false'>Payment History</button></td>\n";
$form .= "<form method='post' action='payee_debts.php'>";
$form .= "<input type='hidden' name='id' value='" . $value['paid'] . "'>\n";
$form .= "<input type='hidden' name='payee_id' value='" . $payee_id . "'>\n";
$form .= "<td style='text-align: center;'><input type='submit' name='delete' value='X'></form></td>\n";
$form .= "<form method='post' action='payee_debts.php'>";
$form .= "<input type='hidden' name='id' value='" . $value['paid'] . "'>\n";
$form .= "<input type='hidden' name='payee_id' value='" . $payee_id . "'>\n";
$form .= "<td style='text-align: center;'><input type='submit' name='editaccount' value='E'></form></td>\n";
$form .= "</tr>\n";
}
}
$form .= "<tr style='text-align: center;'>\n";
$form .= "<td colspan='7'><form class='' method='post' action='payee_debts.php'>\n";
$form .= "<input type='hidden' name='action' value='new'>\n";
$form .= "<input type='hidden' name='payee_id' value='" . $payee_id . "'>\n";
$form .= "<input type='submit' class='btn btn-info' name='submitnew' value='Add Debt'>\n";
$form .= "</form></td>\n";
$form .= "</tr>\n";
$form .= "</table>\n";
$form .= "<!-- Trigger the modal with a button -->";
$form .= "<!-- Modal -->";
$form .= "<div id='myHistory' class='modal fade' role='dialog'>";
$form .= " <div class='modal-dialog modal-lg'>";
$form .= " <!-- Modal content-->";
$form .= " <div class='modal-content'>";
$form .= " <div class='modal-header'>";
$form .= " <button type='button' class='close' data-dismiss='modal'>×</button>";
$form .= " <h4 class='modal-title text-center'>Debt Payment History</h4>";
$form .= " </div>";
$form .= " <div class='modal-body'>";
$form .= $this->GetDebtHistory('passed id value from button');
$form .= " </div>";
$form .= " <div class='modal-footer'>";
$form .= " <button type='button' class='btn btn-default' data-dismiss='modal'>Close</button>";
$form .= " </div>";
$form .= " </div>";
$form .= " </div>";
$form .= "</div>"; //End of Modal
$form .= "</div>\n";
$form .= "</div>\n";
return $form;
}
6 Answers
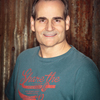
Bill Dowd
7,681 PointsFor the sake of moving on, I came up with an acceptable solution. It may be the only solution, but I don't know. Inside the loop of the main function and inside the <td></td> tags that contain the button, I added all the modal code. Because it's inside the loop, I can directly plug in the debt_id to the function call inside the modal. Because the modal is repeatedly included in the list, I had to append the modal id and data-target, which are supposed to be the same, with the debt_id. The result is "History1" "History2", ... for each loop. Otherwise, the modal call would not work.
The downside is there is extra code in each row of the table. By the way, the reason I added the code inside the <td></td> tags with the button code, was to hide it. I originally had added another row with a single <td></td> set spanning all the columns, but you could see a slightly fatter line between each row. Here is the final function.
// For the Payee Dashboard
public function FormatPayeeDebtsTable($payee_id) {
$objVendor = new Vendor(0);
$form = "";
$form .= "<div class='row'>\n";
$form .= "<div class='col-md-12'>\n";
$form .= "<div class='tabletitle2'>Debts</div>\n";
$form .= "<table class='table-striped table-bordered' width='99%'>\n";
$form .= "<tr>\n";
$form .= "\t<th width='25%'>Vendor</th>\n";
$form .= "\t<th width='25%'>Opening<br />Balance</th>\n";
$form .= "\t<th width='25%'>Balance</th>\n";
$form .= "\t<th width='15%'>History</th>\n";
$form .= "\t<th width='5%'> </th>\n";
$form .= "\t<th width='5%'> </th>\n";
$form .= "</tr>\n";
if(empty($this->GetPayeeDebts($payee_id))) {
$form .= "<tr>\n";
$form .= "<td colspan='6'>There are no debts to display.</td>\n";
$form .= "</tr>\n";
} else {
$results = $this->GetPayeeDebts($payee_id);
foreach($results as $value) {
$this->id = $value['id'];
$this->opening_balance = $value['opening_balance'];
$form .= "<tr>\n";
$form .= "<td>" . $objVendor->GetVendorName($value['vendor_id']) . "</td>\n";
$form .= "<td>$" . number_format($value['opening_balance'], 2) . "</td>\n";
$form .= "<td>$" . ($this->GetDebtBalance($value['id']) == 0 ? number_format($value['opening_balance'], 2) : number_format($value['opening_balance'] + $this->GetDebtBalance($value['id']), 2)) . "</td>\n";
$form .= "<td style='text-align: center;'><button type='button' class='btn btn-info btn-sm' data-toggle='modal' data-target='#History" . $value['id'] . "' data-did='" . $value['id'] . "'>Payment History</button>";
$form .= "<!-- Trigger the modal with a button -->\n";
$form .= "<!-- Modal -->\n";
$form .= "<div id='History" . $value['id'] . "' class='modal fade' role='dialog'>\n";
$form .= " <div class='modal-dialog modal-lg'>\n";
$form .= " <!-- Modal content-->\n";
$form .= " <div class='modal-content'>\n";
$form .= " <div class='modal-header'>\n";
$form .= " <button type='button' class='close' data-dismiss='modal'>×</button>\n";
$form .= " <h4 class='modal-title text-center'>Debt Payment History for " . $objVendor->GetVendorName($value['vendor_id']) . "</h4>\n";
$form .= " </div>\n";
$form .= " <div class='modal-body'>\n";
$form .= $this->GetDebtHistory($value['id']);
$form .= " </div>\n";
$form .= " <div class='modal-footer'>\n";
$form .= " <button type='button' class='btn btn-default' data-dismiss='modal'>Close</button>\n";
$form .= " </div>\n";
$form .= " </div>\n";
$form .= " </div>\n";
$form .= "</div>\n"; //End of Modal
$form .= "</td>\n";
$form .= "<form method='post' action='payee_debts.php'>";
$form .= "<input type='hidden' name='id' value='" . $value['id'] . "'>\n";
$form .= "<input type='hidden' name='payee_id' value='" . $payee_id . "'>\n";
$form .= "<td style='text-align: center;'><input type='submit' name='delete' value='X'></form></td>\n";
$form .= "<form method='post' action='payee_debts.php'>";
$form .= "<input type='hidden' name='id' value='" . $value['id'] . "'>\n";
$form .= "<input type='hidden' name='payee_id' value='" . $payee_id . "'>\n";
$form .= "<td style='text-align: center;'><input type='submit' name='editdebt' value='E'></form></td>\n";
}
}
$form .= "<tr style='text-align: center;'>\n";
$form .= "<td colspan='7'><form class='' method='post' action='payee_debts.php'>\n";
$form .= "<input type='hidden' name='action' value='new'>\n";
$form .= "<input type='hidden' name='payee_id' value='" . $payee_id . "'>\n";
$form .= "<input type='submit' class='btn btn-info' name='submitnew' value='Add Debt'>\n";
$form .= "</form></td>\n";
$form .= "</tr>\n";
$form .= "</table>\n";
$form .= "</div>\n";
$form .= "</div>\n";
return $form;
}
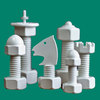
Steven Parker
229,785 PointsI seems like you could use a dataset attribute for this.
What if you add another dataset attribute, something like "data-resultid
"? Wouldn't that do?
Also, what language is this? It doesn't look like JavaScript!
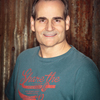
Bill Dowd
7,681 PointsHi Steven,
Thank you for your response. It is PHP, but my weakness is Javascript, which I'm trying to integrate more into the application where it will be a benefit. The function pulls the debts for the person out of a database and displays them in a table. Because there could be more than one debt, I need to have the value of the debt_id embedded in each button (data-debt_id). The button opens the modal for the corresponding debt and displays the history of payments for that debt, starting balance and ending balance. It does that by calling another function to assemble the data and table for the history.
I have figured out the "data-resultid" part and I believe I have to use Javascript to process the button and get the data-debt_id, but I don't know how to get it into the function call in the modal.
Other than the button code, I'm unclear on the Javascript that will handle the button and I'm completely lost on how to get the "debt_id" to the PHP function call inside the body of the modal. I have spent time enjoying the many Javascript, JQuery, and AJAX related lessons on this site, but there is so much to understand and too many different ways to accomplish the same task. Often, too many ways prevents learning one good way and letting the development of learning other ways to happen after mastering the first.
The results I have so far are so awesome that I don't want to substitute another way. I can visualize this same solution into other areas of the application. So success is very desirable. Thanks, Bill
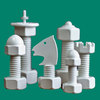
Steven Parker
229,785 PointsIt may be that what you want is happening in the wrong tier.
I'm a full-stack developer, but not PHP-savvy. But if I understand correctly, your PHP code runs in the server before the page is rendered to the user. So it doesn't seem like you could get data that is only determined when the user presses a button on the rendered page into the modal code at the server before the page is rendered.
Or are you looking for some JavaScript code to postback a request to the server when the button is pressed and then have the server deliver something back to the browser?
Alternately, if you're prepared to render all possible results in the modal, but make them hidden by default, you could have some JavaScript triggered by the button reveal the appropriate data when the modal is displayed.
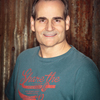
Bill Dowd
7,681 PointsTrue, PHP runs on the server, but what's happening is when the data along with HTML and CSS is sent to the user, it includes the debt_id for each result. The JavaScript then can take that debt_id, which would be different for each selection in the list, and feed it to the function call inside the modal. No need for JavaScript to postback anything to the server as the function call will take care of that. I'd rather not have to render all possible debt results even though in most cases, the payee will only have one. However, that is an option that I might have to resort to.
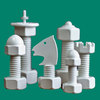
Steven Parker
229,785 PointsI'm a bit confused when you say, "The JavaScript then can take that debt_id, which would be different for each selection in the list, and feed it to the function call inside the modal."
Isn't that function call you're talking about part of the PHP code that is executed at the server before the page is delivered to the client?
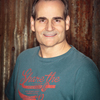
Bill Dowd
7,681 PointsThe page would be rendered with the debt_id as a number producing part of the button, data-5 and for the next record, data-8 and so on. When the button is pressed, the number 5 or 8, depending on which button is pressed, would send the number 5 to the modal where the PHP function needs to have the number 5 inside its parenthesis. The function returns the formatted table to the modal body which in turn, presents it to the user. Does that help?
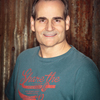
Bill Dowd
7,681 PointsHere is a "view source" sample of the results from this function.
<tr>
<td>Bubba's Stuff</td>
<td>$350.00</td>
<td>$684.00</td>
<td style='text-align: center;'><button type='button' class='btn btn-info btn-sm' data-toggle='modal' data-target='#myHistory' data-did='1'>Payment History</button></td>
<form method='post' action='payee_debts.php'><input type='hidden' name='id' value=''>
<input type='hidden' name='payee_id' value='82'>
<td style='text-align: center;'><input type='submit' name='delete' value='X'></form></td>
<form method='post' action='payee_debts.php'><input type='hidden' name='id' value=''>
<input type='hidden' name='payee_id' value='82'>
<td style='text-align: center;'><input type='submit' name='editaccount' value='E'></form></td>
</tr>
<tr>
<td>Best Buy</td>
<td>$1,250.00</td>
<td>$1,250.00</td>
<td style='text-align: center;'><button type='button' class='btn btn-info btn-sm' data-toggle='modal' data-target='#myHistory' data-did='2'>Payment History</button></td>
<form method='post' action='payee_debts.php'><input type='hidden' name='id' value=''>
<input type='hidden' name='payee_id' value='82'>
<td style='text-align: center;'><input type='submit' name='delete' value='X'></form></td>
<form method='post' action='payee_debts.php'><input type='hidden' name='id' value=''>
<input type='hidden' name='payee_id' value='82'>
<td style='text-align: center;'><input type='submit' name='editaccount' value='E'></form></td>
</tr>
Here is the modal.
<!-- Modal -->
<div id='myHistory' class='modal fade' role='dialog'>
<div class='modal-dialog modal-lg'>
<!-- Modal content-->
<div class='modal-content'>
<div class='modal-header'>
<button type='button' class='close' data-dismiss='modal'>×</button>
<h4 class='modal-title text-center'>Debt Payment History</h4>
</div>
<div class='modal-body'>
There is no history to display. </div>
<div class='modal-footer'>
<button type='button' class='btn btn-default' data-dismiss='modal'>Close</button>
</div>
</div>
</div>
</div>
Notice the text "There is no history to display" is due to the lack of an id getting from the button to the modal.
I came across this code to "activate" the modal. I believe this would be the place where the data-did=1 would be processed and sent to the modal???
<script>
$(document).ready(function(){
$("#myBtn").click(function(){
$("#myModal").modal();
});
});
</script>
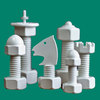
Steven Parker
229,785 PointsOne of us is missing something, because I have the same confusion. You said, " When the button is pressed, the number 5 or 8, depending on which button is pressed, would send the number 5 to the modal where the PHP function needs to have the number 5 inside its parenthesis. "
But that PHP function you say needs to have the chosen number in parentheses .. wasn't that function already executed at the server before the page was transmitted?
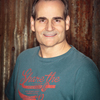
Bill Dowd
7,681 PointsNo. What happens is the function inside the modal makes another call to the server and returns the results to the modal. The base function, the one at the top of this post, returns the code in the "view source" above. Then when the button is pressed, JavaScript does its thing which results in a PHP function call inside the modal, which then makes the call to the server and returns the formatted table to the modal.
If I hard code in an id number to the function inside the modal, it works beautifully.
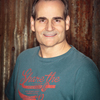
Bill Dowd
7,681 PointsThanks Steven. The bottom line was the button and PHP function call in the modal worked when I coded in an id number. What I was unable to do and what I was hoping to get was how to connect that id from the button to the PHP function parenthesis. If JavaScript (JQuery, AJAX, etc.) could do it, I was ignorant as to how and unable to guess my way through. Maybe in the future, someone will be able to make that connection for me and help me to understand. Meanwhile, as often as I can, I'll watch and rewatch the plethora of great lessons here on Teamtreehouse.
Steven Parker
229,785 PointsSteven Parker
229,785 PointsThat's the only way I'd expect you could do it from the client side. I admit to not knowing PHP, but I sure didn't see anything that remotely looked like it would generate a server postback and then update the page on response.
To eliminate the "fattness" you'd need to control the visibility of the table cell itself and not just the modal inside it.