Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial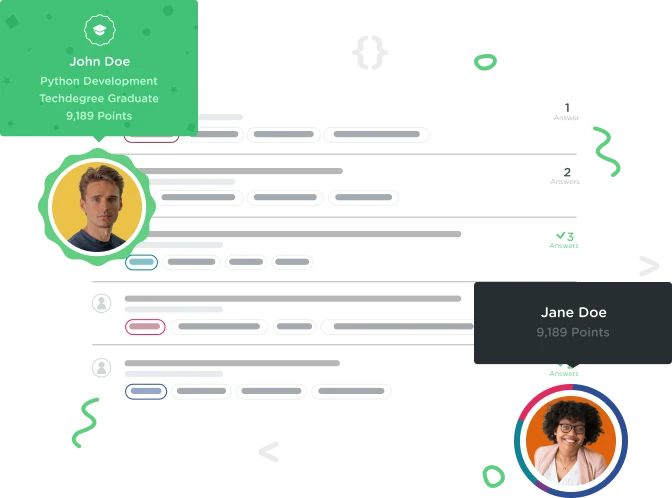
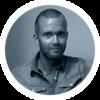
Daniel Fitzhugh
9,715 PointsHow to Post Data using Regular Javascript
FYI those who want to know how to post data (as shown in this video) but with regular javascript....
I personally don't like learning stuff in jQuery without knowing how to do it regular javascript.... so I read a couple of articles (this one was particularly useful: https://developer.mozilla.org/en-US/docs/Learn/HTML/Forms/Sending_forms_through_JavaScript) and worked it out.
Any problems with this code? As you can see, it's ridiculously long compared to the jQuery version:
const form = document.querySelector("form");
form.addEventListener("submit", function (event) {
// TAKE BACK DEFAULT BEHAVIOURS FROM THE HTML FORM
event.preventDefault();
const url = form.getAttribute("action");
// GATHER UP FORM DATA INTO A SINGLE QUERY STRING
const formElements = document.querySelectorAll("form input, form textarea, form select");
const arrayForString = [];
for ( let i = 0; i < formElements.length; i += 1 ) {
if ( formElements[i].hasAttribute("name") ) {
const element = formElements[i];
const name = element.getAttribute("name");
const value = element.value;
const nameValue = encodeURIComponent(name) + "=" + encodeURIComponent(value);
arrayForString.push(nameValue);
}
}
const formData = arrayForString.join("&");
// CREATE THE XHR REQUEST
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if ( xhr.readyState === 4 && xhr.status === 200 ) {
document.querySelector("#signup").innerHTML = "<p>Thanks for signing up!</p>";
}
}
xhr.open("POST", url);
xhr.send(formData);
});
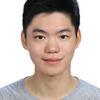
Ga In Shin
9,930 Pointsfantastic you!
3 Answers
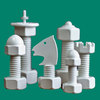
Steven Parker
231,269 PointsAnd now you know why jQuery is so popular for using AJAX.
Another advantage is that the jQuery methods resolve certain CORS security access issues that would take even more code (or the use of a CORS proxy) to handle.

Nour El-din El-helw
8,241 PointsSo, I tried to do the same thing but using the fetch api..
document.querySelector('form').addEventListener('submit', e => {
e.preventDefault();
const formElements = document.querySelectorAll('input[type=text]', 'input[type=email]');
let encodedFormElements = [];
for( let i =0; i < formElements; i++ ) {
encodedFormElements.push(
encodeURIComponent(formElements[i].getAttribute('name')) + '=' +
encodeURIComponent(formElements[i].value));
}
let encodedData = encodedFormElements.join('&');
const data = {
method: 'POST',
body: encodedData
}
fetch(this.action, data)
.then(() => {
document.querySelector('#signup').innerHTML = '<p>Thanks for signing up!</p>';
})
})
but for some reason when I try to console.log(encodedData) it logs white space
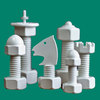
Steven Parker
231,269 PointsI haven't tried this out but one thing you might consider is that while "fetch" provides a greatly simplified interface, it also does not resolve CORS issues the way jQuery does.

Nour El-din El-helw
8,241 PointsYeah, everything has it's pros and cons. I just did so because at the end of the fetch api workshop,Guil told us to remake all our projects that use xhrhttpobject and jQuery for ajax with fetch api, and to be honest it's kind of fun too. xD
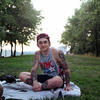
Jake Goldstein
1,304 PointsThanks man I bookmarked this because I agree I like to at least see how things are done in vanilla javascript when learning frameworks or new libraries
Masha Blair
13,005 PointsMasha Blair
13,005 PointsThank you so much for preparing this, Daniel!