Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial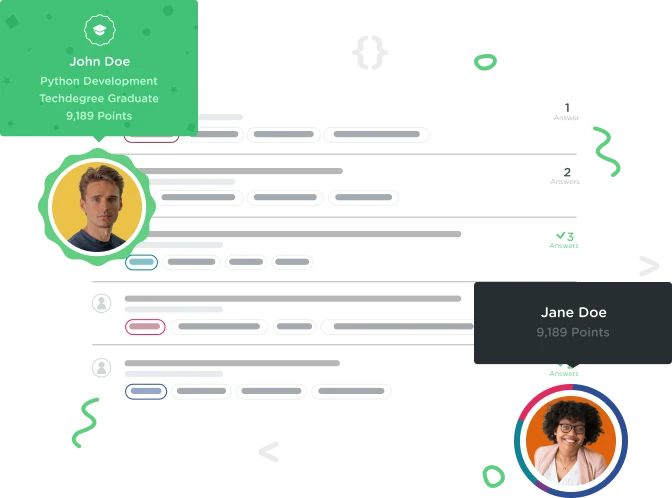

Amir Gamil
5,657 PointsHow to prevent a delegate method running?
I am extending the weather app that we had to build in the networking programming course. I have implemented location detection however, I am tying to add a settings view controller where the user can change his/her location there. Every time my initial view controller loads, it runs a delegate method which sets the coordinate value. So how would you change the coordinate value from a different view controller if it automatically sets it in the previous controller. My code for my main view (view did load method is shown below:
override func viewDidLoad() {
super.viewDidLoad()
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestWhenInUseAuthorization()
locationManager.startUpdatingLocation()
}
I have tried setting the delegate in the prepageForSeuge as nil however every time the view loads again, it just resets it. Any idea how I could solve this?
2 Answers
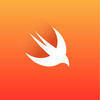
Steven Deutsch
21,046 PointsHey Amir Gamil,
You're going to want to do a few things. First would be to remove start updating location from viewDidLoad. You only want to call this method if the user has not set a specific location for the app to use. I would try something simple at first, maybe using NSUserDefaults.
/// When a user sets a latitude/longitude in settings, store them in the NSUserDefaults using a key
NSUserDefaults.standardUserDefaults.setValue(20, forKey: "longitude")
NSUserDefaults.standardUserDefaults.setValue(40, forKey: "latitude")
/// Then in viewDidLoad you can check
func locationIsSet() -> Bool {
let lon = NSUserDefaults.standardUserDefaults.valueForKey("longitude")
let lat = NSUserDefaults.standardUserDefaults.valueForKey("latitude")
return lat != nil && lon != nil
}
if locationIsSet {
/// use locations from defaults
locationManager.endUpdatingLocation()
} else {
/// monitor for location
locationManager.startUpdatingLocation()
}
I don't know if I'm using the proper method names but hopefully this gives you a general idea. There are many ways to go about this. Good Luck!

Amir Gamil
5,657 PointsThanks for your help! I'd already found a solution but this could be a way to refactor my current code. :)