Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial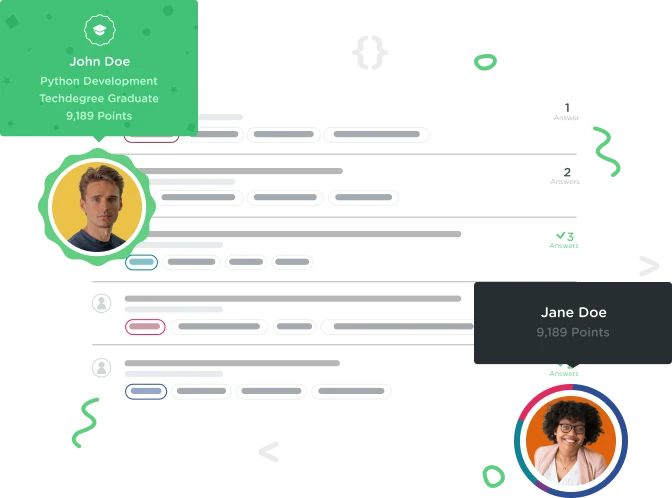
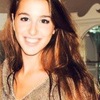
Sonia Feldman
3,873 PointsHow to Prevent Fact Repetition
Hi,
I was wondering if anyone knew a simple way to prevent the app from showing the same fact twice in a row or, rather, to ensure that every fact shown will be different from the fact that appeared previously.
I know this isn't necessary for the app to work, and that with a larger base of facts stored in the FactModel repetition would become less and less likely, I am just curious.
3 Answers

Anjali Pasupathy
28,883 PointsSomeone else asked a similar question regarding the background color. I provided a quick solution, and you could probably do something similar to prevent yourself from repeating fun facts.
https://teamtreehouse.com/community/non-repeating-random-number-with-uicolor
I hope that helps!
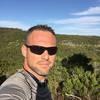
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsHere is how I figured out how to do it. This way they don't repeat until all facts have been cycled through and then it just resets.
func randomSequenceGenerator(min: Int, max: Int) -> () -> String {
var numbers: [Int] = []
return {
if numbers.count == 0 {
numbers = Array(min ... max)
}
let index = Int(arc4random_uniform(UInt32(numbers.count)))
return self.facts[numbers.remove(at: index)]
}
}
Then in ViewController change your factProvider. Make sure to set it from 0 to the count minus 1 as the first item in the array is 0.
let factProvider = StrangeFacts().randomSequenceGenerator(min: 0, max: StrangeFacts().facts.count - 1)
Lastly in the ViewController you need to change funFactLabel.text in both the viewDidLoad as well as the IBAction.
funFactLabel.text = factProvider()
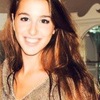
Sonia Feldman
3,873 PointsThank you!

Anjali Pasupathy
28,883 PointsYou're welcome!