Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial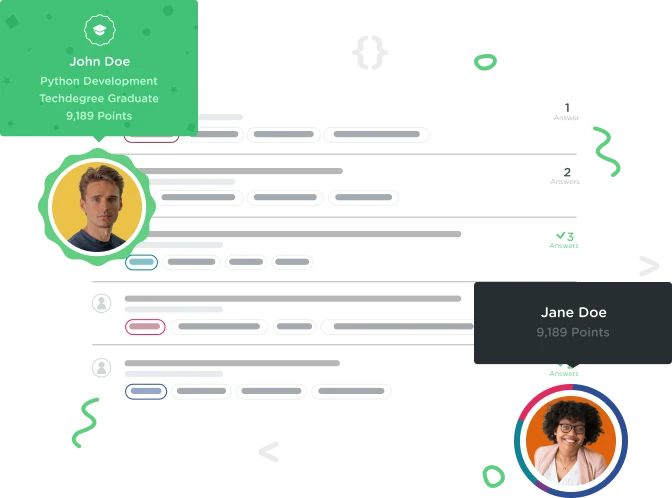

E. T.
Python Development Techdegree Student 884 PointsHow to print inventory as a list rather than printing its items one at a time?
how to write a method for that?
3 Answers
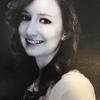
Megan Amendola
Treehouse TeacherI can help better if you post your code E.T.
When I use the code I wrote above it works fine:
class Inventory:
def __init__(self):
self.inventory = []
def get_inventory(self):
return self.inventory
def add_item(self, item):
self.inventory.append(item)
inventory = Inventory()
inventory.add_item('grapes')
inventory.add_item('bananas')
print(inventory.get_inventory())
---Console---
['grapes', 'bananas']
If you're getting object locations, then I am assuming your list is filled with objects of another class. Something like this:
class Inventory:
def __init__(self):
self.inventory = []
def get_inventory(self):
return self.inventory
def add_item(self, item):
self.inventory.append(item)
class Item:
def __init__(self, item):
self.item = item
def __str__(self):
return self.item
def __repr__(self):
return self.item
inventory = Inventory()
inventory.add_item(Item('grapes'))
inventory.add_item(Item('bananas'))
# this uses __repr__
print(inventory.get_inventory())
# this uses __str__
for item in inventory.inventory:
print(item)
---Console---
[grapes, bananas]
grapes
bananas
That means you need to add a __str__
method to access each item in your list. If you want to see your list print to the console, then you'll need a __repr__
. Here's some more detail on the two. Print a list works differently than just printing out an individual object.
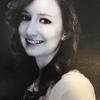
Megan Amendola
Treehouse TeacherHi, E. T.
You can add a method to your class to print()
or return
the list depending on what you want to do with it. For example:
class Inventory:
def __init__(self):
self.inventory = []
def get_inventory(self):
return self.inventory

E. T.
Python Development Techdegree Student 884 PointsMegan Amendola Still not getting it. I want to print the list. I tried after adding get_inventory() method:
>>> inventory.slots
<items.Item object at [address] >, <items.Item object at [address]>
It's giving me the addresses rather than showing what items are in that list!

E. T.
Python Development Techdegree Student 884 PointsMegan Amendola Thanks a ton :)
If you want to see your list print to the console, then you'll need a
__repr__
.....Print a list works differently than just printing out an individual object.
This helps.