Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial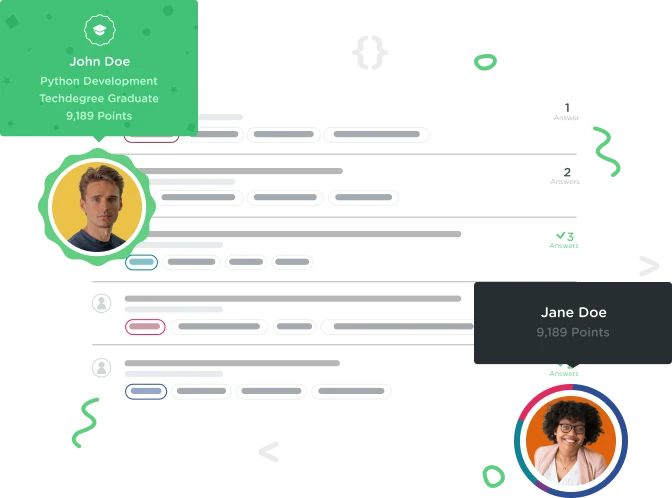
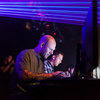
Adam Duffield
30,494 PointsHow to print mysql database data to the website?
Hi,
This is my php/mysql code for trying to get data from the database and print it to the screen to show the ID, Title & Info in the database.
try { $db = new PDO("mysql:host=localhost;dbname=MyBlog;port=3306","root",""); $db->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION); } catch (Exception $e) { echo "Could not connect to the database."; exit; }
try { $results = $db->query("SELECT 'ID','Title','Info' FROM myblog"); } catch(Exception $e) { echo "Data could not be retrieved from database" . $e; exit(); }
$products = $results->fetchAll(PDO::FETCH_ASSOC);
echo $products;
The website shows this following error, any ideas how i can convert this to show the actual results?
Notice: Array to string conversion in C:\xampp\htdocs\Projects\Portfolio\Model\database.php on line 29 Array
Thanks,
Adam
4 Answers
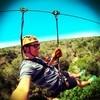
thomascawthorn
22,986 PointsThe error message here is quite descriptive. You've created an array and stored it into the variable $products.
Unfortunately, you can't echo an array. You can check the contents by:
<?php
echo '<pre>'; //html tag for nice format!
var_dump($array);
exit;
If you want to pull out the information in turn, you can use a foreach loop.
<ul>
<?php
foreach ($products as $product) {
echo '<li>';
echo $product['Title'];
echo $product['Info'];
echo '</li>';
}
?>
</ul>
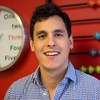
Nick Ocampo
15,661 PointsYou're trying to print an array. Try echo serialize($products);
That will return the data in your array as a string.
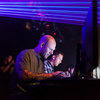
Adam Duffield
30,494 PointsHey thanks for the quick reply! I tried that but I get this results instead of an ID of 0 a title of my 1st blog and some random info.
a:1:{i:0;a:3:{s:2:"ID";s:2:"ID";s:5:"Title";s:5:"Title";s:4:"Info";s:4:"Info";}}
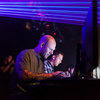
Adam Duffield
30,494 PointsHey,
Thanks for the comments both of you but It's still not printing out what I'm after, it just prints that I have an array with the titles of ID, Title and Info but not the actual ID, Title and Info details
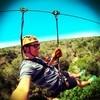
thomascawthorn
22,986 Pointscan you run
<?php
echo '<pre>'; //html tag for nice format!
var_dump($array);
exit;
and post the result?
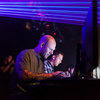
Adam Duffield
30,494 Pointshey sorry for the late reply, these are the results...
object(PDOStatement)#2 (1) { ["queryString"]=> string(38) "SELECT 'ID','Title','Data' FROM myblog" }
Any ideas?
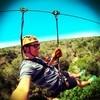
thomascawthorn
22,986 PointsInstead of $array, did you put $results or $products?