Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial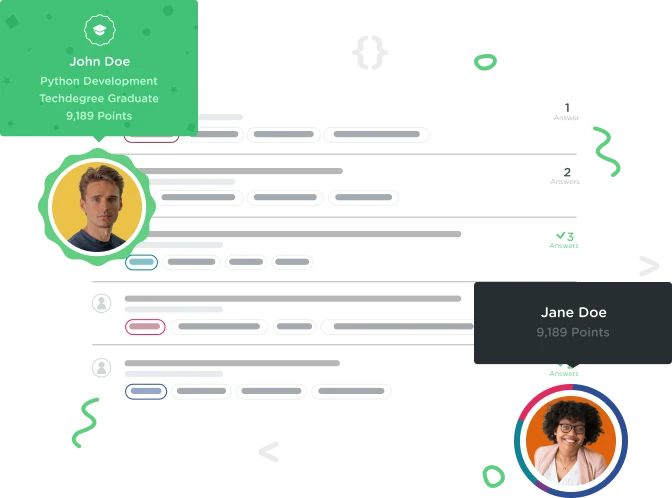

Devin Rayner
14,650 PointsHow to produce a menu using the range function?
I have been working on this problem for quite some time and I just can't figure it out?
1 Answer

Michael Switzer
7,490 PointsThis was a real struggle for me too. Here is what I finally ended up with:
public static List<String> getStateCodesFromRecords(List<HousingRecord> records) {
return records.stream()
// TODO: Map the stream to the state code
.map(HousingRecord::getState)
// TODO: Filter out any records without a state
.filter(state -> !state.isEmpty())
// TODO: There are duplicate state codes in the records, make sure we have a unique representation
.distinct()
// TODO: Sort them alphabetically
.sorted()
// TODO: Collect them into a new list.
.collect(Collectors.toList());
}
public static void displayStateCodeMenuDeclaratively(List<String> stateCodes) {
// TODO: Use a range to display a numbered list of the states, starting at 1.
IntStream.rangeClosed(1, stateCodes.size())
.mapToObj(count -> String.format("%d. %s", count, stateCodes.get(count - 1)))
.forEach(System.out::println);
}